Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial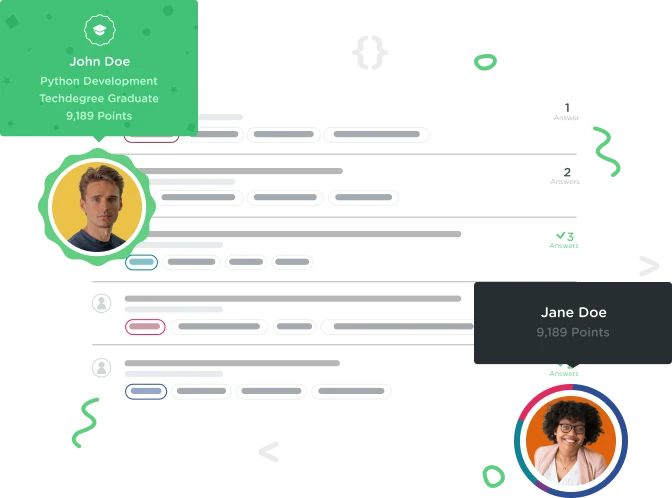
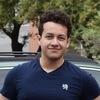
James Estrada
Full Stack JavaScript Techdegree Student 25,867 PointsWhat's the difference between a variable and an object?
For example, in a quiz question it says: "Bob" is an __ of type string. Where object is the right answer. This makes me question where's the difference between a variable and an object. From the previous example the string can be formed this way:
string name = "bob";
so bob is the object, string is the type, and name is the variable.
Now, throughout the course, when we built a tower object, what's the object in here:
Tower tower = new Tower();
I can think that the first "Tower" is the type, and "tower" is the variable name, but where is the object? Isn't "new Tower()" creating the object? Or is the variable "tower" the object?
3 Answers

Roman Fincher
18,267 PointsThe short answer is: it's complicated. I think the quickest way to clarify your confusion though is that in a sense, everything is an object. The string type and the Tower type both inherit from System.Object (see MSDN System.String under the inheritance hierarchy at the top).
Strings are just so common that we have a shortcut to instantiate a string object, but "bob" is an object nonetheless. name is not the variable, but rather a reference to the object "bob" (which is an instance of the System.String type). As far as I can tell, there may not actually be a concrete definition for a "variable", at least in this context.
Also consider that you COULD instantiate an instance of the string object and assign it to name by calling:
string name = new string(new char[] { 'b', 'o', 'b' });
when you type name, say in an interactive C# window, it will return "bob", a string. Here we created an instance of the string type by passing a character array (also an object) into one of the string type's constructor overloads. However, because doing it this way is unnecessarily complicated, we can just say string name = "bob" (or even var bob = "bob") because the " " tell the compiler we want a string. It's worth noting that integers are also objects of Type Int.32, which also inherits from System.Object.
As for the tower, "tower" is a reference to an instance (an object) of the Tower type. "new Tower()" is creating an instance (which, again is an object, but there can be many instances of the same type of object, so it's worth being picky about terms). "tower" is not a variable or the object, but a reference used to find the particular object of type Tower in memory.
Variables don't necessarily have a separate meaning because they would all still be objects. However, if something is likely to take on different "values" within the code, it might be convenient to refer to it as a variable to communicate that. This raises another black hole of questions though, because there's also a difference between reference and value types. I'd look at this link for some info on that, but it would still be incorrect to assume variable means value type and object means reference type, because this has to do with how data is stored in memory.
Hope that helps a little bit! I'm no expert and I may be mistaken myself (please feel free to correct me), but It's a really great question!

Mohammed Ajaz
5,542 PointsI think a variable is just storing data like putting something in a box, think of the box as a variable. The object is a created thing from it's class template, it has behaviours and attibutes and it can interact with things.
the variable on the other hand can just store data.
correct me if I'm wrong :)

Christopher Debove
Courses Plus Student 18,373 PointsSimply speaking the object in your second portion of code is "new Tower()", more precisely new Tower()
returns an object (instance of) Tower.
So in this code:
Tower tower = new Tower();
Your creating a variable tower, of type Tower for which you assign a new instance of Tower.