Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial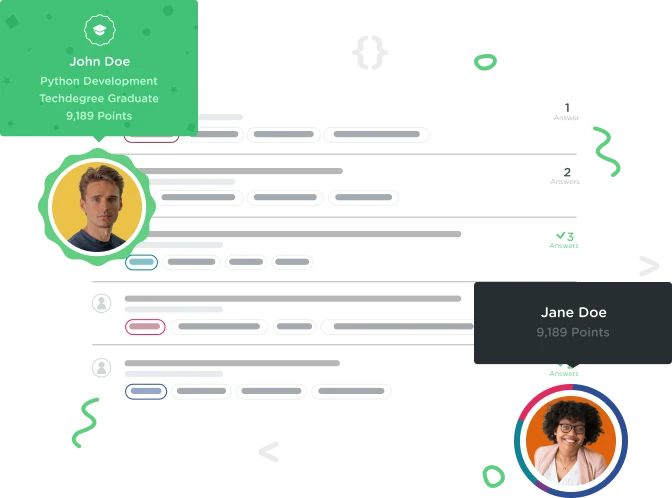

brandonlind2
7,823 PointsWhats the difference between an event listener and an event handler?
Is there a difference between the two, if so can someone give me an example on the differences between the two?
3 Answers
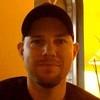
Jeremy Hill
29,567 PointsEvent listener basically waits for the call for the event and then notifies the handler when to initiate it.

brandonlind2
7,823 Pointscould you give me an example of a listener, for example if I was writing javascript and wrote element.onclick=function(){} what part of this would be the listener or is the listen some default method that you dont normally work with when writing code? I'm assuming the above thing is the handler?

Filip Kaniski
6,677 PointsThink about the names and what they mean, that's how it first clicked for me. Event listener "listens" for an event, and an event handler "handles" it.
To use an example you put down:
element.onclick=function(){}
How do you know what event this code listening for? .onclick right?
And when it hears a click, how does it handle the event? Because there's not much point to listening for an event if you don't do anything about it right? Well there's a function that tells it exactly what to do right next to it.
Also, just to elaborate a bit further, remember that using your syntax you can only bind one handler to an onclick event. If you tried to add .onclick = someotherfunction later on in your code, the initial handler would no longer be run when a click is registered. To run multiple handlers on a single event, use the .addEventListener() method. Look up the documentation for syntax, but it takes a listener and a handler as arguments.
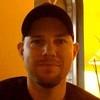
Jeremy Hill
29,567 PointsWell I know that in java you have to implement the listener; I'm not sure about javascript as I still have yet to study it more. But the listener is waiting for something to be clicked as in your example above; when the click takes place it tells the handler to do something, whatever the function may be. I'm not real sure about javascript but if I had to guess I would say that it is built in.
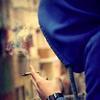
Tushar Singh
Courses Plus Student 8,692 PointsNo difference. Check this out
paulca55
7,284 Pointspaulca55
7,284 PointsOne difference is that if you add two event handlers for the same button click, the second event handler will overwrite the first and only that event will trigger. For example:
But if you use
addEventListener
instead, then both of the triggers will run.Hope this makes sense.