Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial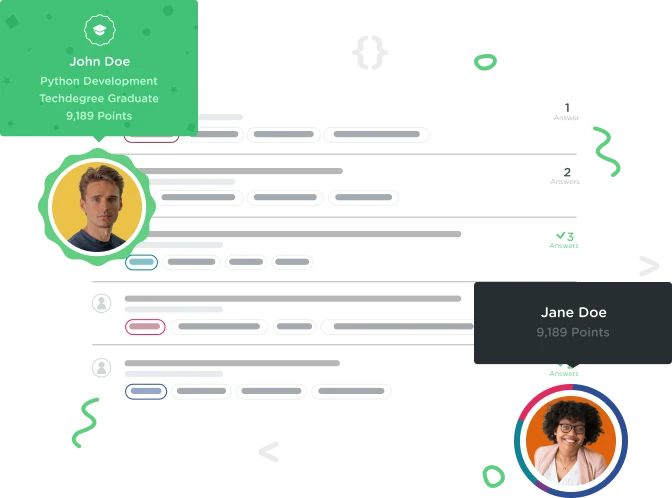
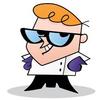
Dr.P reon
8,562 Pointswhats the difference between =, ==, and === ? and in what situations do we use them?
I'm trying to do the extra credit problem on Intro to Programming in the Control Structures section.
The Extra Credit is called Fizz Buzz
Fizz Buzz
Write a program that loops through the numbers 1 through 100. Each number should be printed to the console, using console.log(). However, if the number is a multiple of 3, don't print the number, instead print the word "fizz". If the number is a multiple of 5, print "buzz" instead of the number. If it is a multiple of 3 and a multiple of 5, print "fizzbuzz" instead of the number.
I got confused on how to solve the problem, so I googled it. and I found this code
console.log("Before"); for (var i = 0; i<=100;i+=1) { console.log (i)
if (0==i%15){ console.log ("fizzbuzz"); } else if (0==i%3) { console.log("fizz"); } else if (0==i%5) { console.log ("buzz"); } else { console.log(i); }
}
console.log("After");
i don't understand why they used == in the if /else statement and why the statement begins with 0. I will be very grateful if someone can help me...
3 Answers
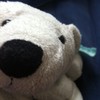
bothxp
16,510 PointsThey are using the Modulus Operator 'Divides the value of one expression by the value of another, and returns the remainder' and checking to see if the result equals 0.
if the number (i) is a multiple of 3, would be i % 3 equals 0.
if the number (i) is a multiple of 5, would be i % 5 equals 0.
if the number (i) is a multiple of 3 & 5 (15) , would be i % 15 equals 0.
Assignment (=), Simple assignment operator which assigns a value to a variable.
Equality (==), The equality operator converts the operands if they are not of the same type, then applies strict comparison. If both operands are objects, then JavaScript compares internal references which are equal when operands refer to the same object in memory.
Identity / strict equality (===), The identity operator returns true if the operands are strictly equal with no type conversion.
I have to admit that the way that they have written if (0==i%15) does look a little odd to me. But it is just checking to see if 0 is equal to i % 15. I would probably have written it the other way around and would have used the strict equality as in if(i%15 === 0){ .... }
and if I was being picky the challenge as you to: "Write a program that loops through the numbers 1 through 100". But that code would loop through the numbers 0 to 100.
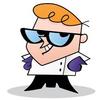
Dr.P reon
8,562 Points@bothxp, yep you are right, i rechecked my work and I made an error in my code. Thank you so much, I am very grateful!

Fidel Torres
25,286 Pointshey I got a new answer hope you like it
//programs starts here
var fizzbuzzArr = new Array();
for(1..... 100){//array from 1 to 100
var fibu = "";
if(i % 3 == 0){
fibu += "fizz"; //if i multiple of 3 add to fibu;
}
if(i % 5 == 0){
fibu += "buzz"; //if i multiple of 5 add to fibu;
}
// just if the i value is divisible by 3 & 5 the var fibu will have the two values in it...
if(fibu != ""){
fizzbuzzArr.push(fibu); // if fibu var isn't empty add to array
/* reference to push function in the link bellow
http://www.w3schools.com/jsref/jsref_push.asp
}
}
// after the loop is finish the rest will be print the values in the array
for(var i = 0 ;i < fizzbuzzArr.length ; i++){ // as we don't know how many values the array has, need to use Arr.length function
console.log(fizzbuzzArr[i]);
}
//programs ends here
Dr.P reon
8,562 PointsDr.P reon
8,562 PointsThank you so much bothxp. I am very grateful :) . I was kind doubtful of the 100. You have really made my day. I am still a begginer and I am trying to understand most of the basics...
I realize that if you start the loop from 1, the multiples of 3 and 5 will be increased by 1, so instead of having (3, 6, 9, 12, 15, 18, 21 etc) you will end up having (4, 5, 10, 13, 16, 19, 22 etc). I guess that is why they used 0 instead of 1
bothxp
16,510 Pointsbothxp
16,510 PointsNo, starting the loop from 1 just means that the 0 value won't be tested by the if statement. The first value of i will be 1. It won't increase the resulting values in the way that you described.