Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial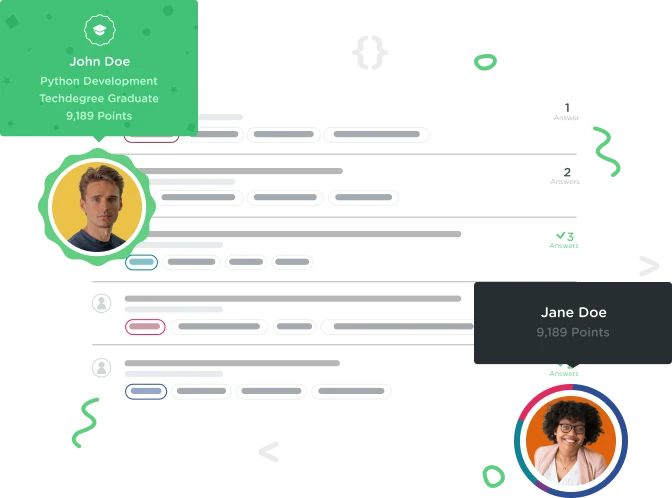
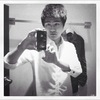
Xing Li
2,112 Pointswhat's the difference between echo and return??
what's the difference between echo and return?
3 Answers

Gunhoo Yoon
5,027 PointsReturn spit out the product of function and anticipates function end.
Echo prints out value to your web page.
<?php
function sayHi() {
return "Hello";
}
sayHi(); //Run sayHi() function. Do no more than that.
$hi = sayHi(); //Assign whatever that is returned by sayHi() to $hi variable.
echo sayHi(); //Print out whatever that is returned by sayHi();
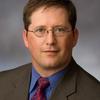
Ted Sumner
Courses Plus Student 17,967 PointsAs noted by Fabio and Gunhoo, echo displays to the screen. Return is very different.
When you have a function (or method in OOP), the code executes within the function but does not send anything out unless you have a return. The return itself does not display anywhere. You can assign it to a variable elsewhere for more to be done to it, you can echo it, or you can just ignore it. For example, you could do this with your function:
<?php
function value() {
$number = 1;
return $number;
}
$val = value();
if ($val !== 1) {
echo "The number is not 1.";
} elseif ($val === 1) {
echo "The number is 1.";
} else {
echo "There was an error. The number is $val.";
}
As you get more into functions you will learn to pass values into the function that will make my example a little more meaningful. But you can see how using return allows you to then analyze the result of the function with more code. When you use databases, you often return an array of values derived from the database. You then need code to process the array into something you can use for the site.
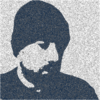
Fábio Tavares da Costa
11,985 Pointsecho prints the value as strings and return calls, for instance a function, and store the value for future use.