Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial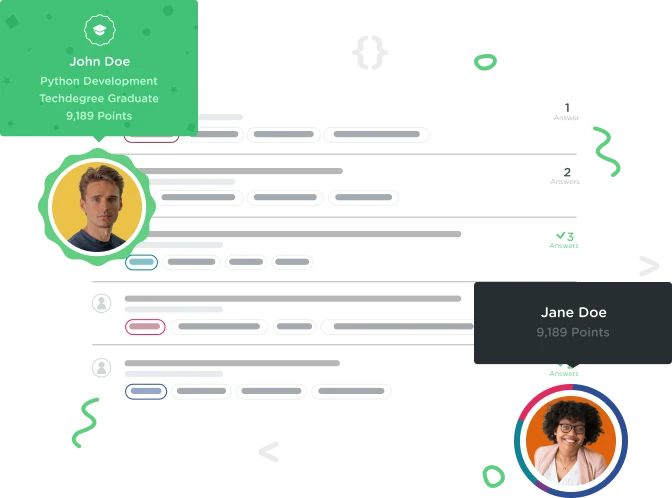

ISAIAH S
1,409 PointsWhat's the difference between the do while block, and the while block?
What's the difference between the do while
block, and the while
block?
2 Answers
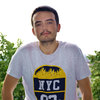
Flamur Beqiri
6,908 PointsThe while statement verifies the condition before entering into the loop to see whether the next loop iteration should occur or not. The do-while statement executes the first iteration without checking the condition, it verifies the condition after finishing each iteration. The do-while statement will always execute the body of a loop at least once.
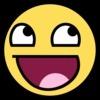
Michael Hess
24,512 PointsHi Isaiah,
A do-while statement is a post-test loop. That means what ever is within the loop's body is executed at least one time. Then if the loop condition is true the loop happens again, if the loop condition is false, the loop is exited.
Here is an example of how a do while loop can be used:
public static void main(String[] args){
int count = 1;
do {
System.out.println("Count is: " + count);
count++;
} while (count < 11);
}
A while loop statement is a pre-test loop -- the loop condition is tested then, if it is true, what ever is within the loop body is executed. If the condition is false the loop is exited.
Here is an example of how a while loop is used:
public static void main(String[] args){
int count = 1;
while (count < 11) {
System.out.println("Count is: " + count);
count++;
}
}
A while loop is also handy for creating an infinite loop to continuously prompt the user for input:
In summary, a while loop tests before the loop and the do/while loop tests after the loop.
Hope this helps!