Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial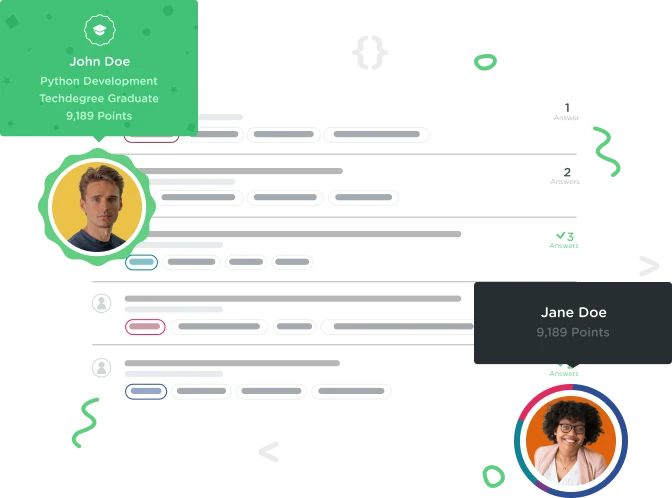
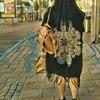
Oshedhe Munasinghe
8,108 PointsWhat's the different between ArrayList and List?
Now I am really confused and I don't think I'm the only one who got mixed.
What is the different between ArrayList and List? I know they are same but not really, or?
Please tell me some examples and tell me step by step instead writing bunch of text!!! Because I'm suffering dyslexia :(
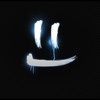
Grigorij Schleifer
10,365 PointsOhhhhh,
forgive me my stupidity
I understood the difference between Array vs. ArrayList not List vs ArrayList ....
Sorry bout that
4 Answers
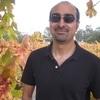
Kourosh Raeen
23,733 PointsAs La Gracchiatrice said above, List is an interface so you cannot write something like:
List<String> myList = new List<String>();
You need to choose one of the implementations of List, like ArrayList or LinkedList:
List<String> myList = new ArrayList<String>();
List<String> myList = new LinkedList<String>();
One consideration is whether to define the list as List or ArrayList. If you do something like:
List<String> myList = new ArrayList<String>();
then you can only call those methods that are in the List interface. So if the ArrayList class has some additional methods, which it does, you cannot call those methods on myList variable. However, if you defined your list as:
ArrayList<String> myList = new ArrayList<String>();
then you can call those addtional methods in ArrayList on myList, in addition to all the ones in the List interface that the ArrayList class has already implemented.
So now the question is why define myList as List<String> ? Why not always define it as ArrayList<String> ? Well, one advantage of defining it as a List is that if at some point you decided to change the implementation of your list from ArrayList to LinkedList then all you have to do is to change the line:
List<String> myList = new ArrayList<String>();
to:
List<String> myList = new LinkedList<String>();
and nothing else needs to change, but if myList was of type ArrayList and you had used ArrayList specific methods then you have to make more changes to your code.
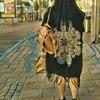
Oshedhe Munasinghe
8,108 Pointssir you made my brain really back to normal thank you so much for hardcoding!
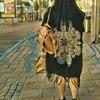
Oshedhe Munasinghe
8,108 PointsGrigorij you are forgiven! :)
La Gracchiatrice Thank you for the information. If you don't mind do you have any code examples? Can't find it in google.. :(
(btw. guys how did you do emoji?)
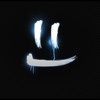
Grigorij Schleifer
10,365 PointsEmojis are cool
//type
:smiley: or :sunglasses:

La Gracchiatrice
4,615 PointsList is an interface that contains only abstract methods, you cannot instantiate an interface (with the new key word), but a List reference can point to an object of any class that implments the List interface (ArrayList, LinkedList ecc) so you can handle any objects of these classes and hide the implemetation of your code.
The BlogsPost and BlogsPot2 examples are similar, they implement a method that returns a List reference so you can change them without change Test and Test2. The difference between BlogsPost and BlogsPot2 is a matter of big O().
The BlogPost3 example implements a method that returns a object of a class that implements List, as Kourosh Raeen said above if you change the implentation of BlogPost3 (eg LinkedList instead of ArrayList ) you have to change the code of Test3.
All classes must be in the same packege
import java.util.Date;
import java.util.List;
public class Test {
public Test(){
}
public static void main(String [] args){
BlogPost blogPost= new BlogPost(
"Author",
"Title",
"oracle site http://www.oracle.com goolge site: https://www.google.com",
"Sites",
new Date()
);
List<String> links=blogPost.getExternalLinks();
for(String aLink:links){
System.out.println(aLink);
}
}
}
import java.util.Date;
import java.util.ArrayList;
import java.util.List;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks(){
ArrayList<String> externalLinks = new ArrayList<>();
for (String aString: getWords()){
if(aString.toLowerCase().startsWith("http")){
externalLinks.add(aString);
}
}
return externalLinks;
}
}
import java.util.Date;
import java.util.List;
public class Test2 {
public Test2(){
}
public static void main(String [] args){
BlogPost2 blogPost= new BlogPost2(
"Author",
"Title",
"oracle site http://www.oracle.com goolge site: https://www.google.com",
"Sites",
new Date()
);
List<String> links=blogPost.getExternalLinks();
for(String aLink:links){
System.out.println(aLink);
}
}
}
import java.util.Date;
import java.util.LinkedList;
import java.util.List;
public class BlogPost2 {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost2(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks(){
LinkedList<String> externalLinks = new LinkedList<>();
for (String aString: getWords()){
if(aString.toLowerCase().startsWith("http")){
externalLinks.add(aString);
}
}
return externalLinks;
}
}
import java.util.Date;
import java.util.ArrayList;
import java.util.List;
public class Test3 {
public Test3(){
}
public static void main(String [] args){
BlogPost3 blogPost= new BlogPost3(
"Author",
"Title",
"oracle site http://www.oracle.com goolge site: https://www.google.com",
"Sites",
new Date()
);
ArrayList<String> links=blogPost.getExternalLinks();
for(String aLink:links){
System.out.println(aLink);
}
}
}
import java.util.Date;
import java.util.ArrayList;
public class BlogPost3 {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost3(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public ArrayList<String> getExternalLinks(){
ArrayList<String> externalLinks = new ArrayList<>();
for (String aString: getWords()){
if(aString.toLowerCase().startsWith("http")){
externalLinks.add(aString);
}
}
return externalLinks;
}
}
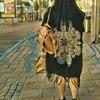
Oshedhe Munasinghe
8,108 Pointswow.. no words.. a big hug.. thank you really much!
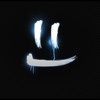
Grigorij Schleifer
10,365 PointsHi Oshedhe,
this is a very important question.
-
Here is a little comparison:
- Cannot change the length of an Array once created. ArrayList can grow by adding elements to it.
- Arrays take less memory then ArrayList for storing same number of objects.
- ArrayList allows you to remove elements, the array not.
- Inside an Array you can accommodate both primitives and objects, inside ArrayList only objects.
- Arrays can be multidimensional. ArrayList is always one dimentional.
Grigorij
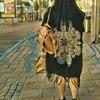
Oshedhe Munasinghe
8,108 PointsHi Grigorij, What do you mean Arrays do you mean List.. :S
So you mean also when I call ArrayList
then I can call method .add .isEmpty() ect. right but if I call List then I cant call .size, .add ect
am I correct?
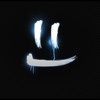
Grigorij Schleifer
10,365 PointsI have to go but I will make another comparison between List and ArrayList ...
Grigorij
La Gracchiatrice
4,615 PointsLa Gracchiatrice
4,615 PointsList is an interface and contains abstract methods. An interface is a contract among developers "I promise to implement methods that behave like these". ArrayList is a class that implements List interface and respects that contract. You can instantiate the class ArrayList with the key word "new" but you can't instantiate the List interface. You can reference an ArrayList object with a List reference eg: List aList = new ArrayList().