Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial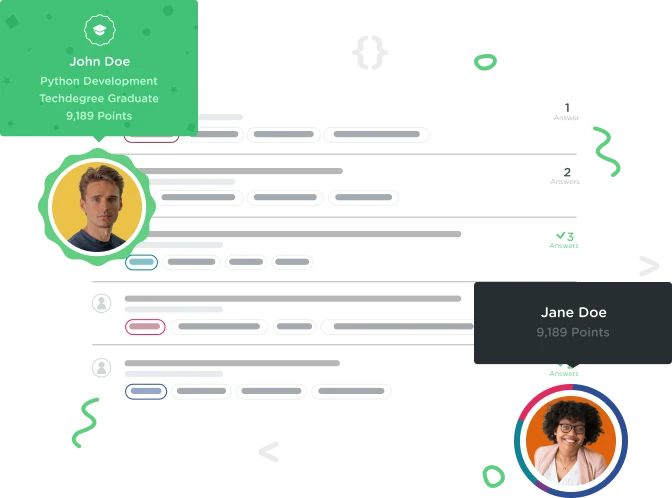
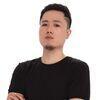
Billy Gragasin
Full Stack JavaScript Techdegree Student 4,985 PointsWhat's the parenthesis for when Guil called the "value" on "const color = `${value()}`?
As far as I understand, you use parenthesis to call a function or method.
But "value" here is inside a declaration statement.
const randomRGB = (value) => {
const color = ${value()}, ${value()}, ${value()}
;
return color;
}
So, it isn't a function? My guess is that you use parenthesis when you want that argument to hold data/value? I need to have a better understanding to this before I can properly sleep!(lol)Thanks, Teamtreehouse! :)
6 Answers
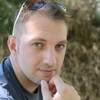
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsMitchell North I am going to break it down a little maybe that will help first off you get a few ways to write a function.
function sayHello(){
console.log('hello')
};
Then you get arrow functions which are being used here
const someName= (someVariable)=>{
console.log(someVariable)
};
syntactically the arrow function is a bit of shorthand
function(a,b){
console.log( a + b);
};
//is the same as
(a,b)=>{
console.log(a+b);
}
//and if there's one argument and statement even shorter
x=> console.log(x);
Heres a course on arrow functions from Guil arrow functions
and heres a link for template literals as well ${value}
Template literals
If you need help with something more specific you will need to give me a less general question. Hope this helped. If it resolved your question mark best answer to mark this as resolved.

Meta Emilie Ünal Hansen
13,338 PointsAs I can see that this question has not been answered and that it's something I would like to know as well, I will try and ask as well.
What I find confusing in the code is why one needs to put the parentheses in the template literals holding the value inside the function = ( ${value()}). When this is usually used to call a function, but here it's used as a value in the function.
Why it looks like this:
function randomRGB (value) {
const color = rgb(${value()}, ${value()}, ${value()})
;
return color;
}
And not this:
function randomRGB (value) {
const color = rgb(${value}, ${value}, ${value})
;
return color;
}
Hope this makes sense.
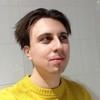
Mitchell North
6,353 PointsI'd like to know the answer to this too. It's a bit confusing using parentheses and I don't think Guil explains their purpose? It's very very likely I missed something but at this early stage I'd like to know...
Also, the code at the end of the video is this:
let html = '';
const randomvalue = () => Math.floor(Math.random() * 256);
function randomRGB = (value) => {
const color = `rgb( ${value()}, ${value()}, ${value()} )`}
return color;
}
for (let i = 1; i <= 10; i++) {
html += `<div style="background-color: ${randomRGB(randomValue)}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;
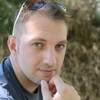
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsBilly Gragasin , could you pop a link to the specific exercise you are speaking about so that this can be shown in the context of the exercise. Without all the info its a little harder to tell.
My assumption is that you are referring to this exercise https://teamtreehouse.com/library/the-refactor-challenge-duplicate-code
So the code you have here a little different to what Guil has, your code shows as follows :
const randomRGB = (value) => { const color = ${value()}, ${value()}, ${value()}; return color; }
where the actual code is meant to be more like this :
const randomRGB = ( ) => { const color = `rgb(${value()}, ${value()}, ${value()}; return color)`; }
Notice here that there is no value argument passed into the arrow function. This is what was confusing you. Your code is also missing the rgb in front of the values which tells you that the 3 values are intended as color values. Also you are missing back ticks before and after to make the template literal work. or alternatively you could use :
function randomeRGB(){
const color = `rgb(${value()}, ${value()}, ${value()})`;
return color;
}
Watch this for an explanation of template literals. link-> template literals
If this has helped solve your question please select best answer to mark this as resolved.
Cheers, Doron
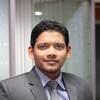
Md. Syful Islam
9,463 PointsBilly Gragasin , As far I have understood, here value()
is just working as an argument to the function randomRGB()
as Guil said, function can be used as an argument to another function. Here, randomRGB()
function expects an argument which will be function.
In the for loop the randomValue
function is passed as an argument to the randomRGB()
function.
value()
is just a place holder.

Zoltán Erkel
6,340 PointsHey there,
I'm pretty sure the () are needed as it will call "randomValue" which is a function, thus it will be called by having parenthesis next to it.
So imo it works as such: value is the parameter inside of the randomRGB() function, the parameter then get's called inside of the "rgb()" property inside the color variant. But since the argument later in the randomRGB() function is the randomValue() function (which is first written as an arrow function), when it gets called inside the rgb, itt will need the parenthesis, so that is runds the Math equasion.
The code actaully looks like this:
let html = '';
const randomValue = () => Math.floor(Math.random() * 256); /*here is the randomValue function created*/
function randomRGB(value) {
const color = `rgb( ${value()}, ${value()}, ${value()})`; /*here he calles the value parameter*/
return color;
}
for (let i=1; i<=100; i++) {
html += `<div style="background-color: ${randomRGB(randomValue)}">${i}</div>` /*Here he adds the randomValue() function as an argument, which then gets inserted into the randomRGB function, thus the () are needed after the value paramater gets called for it to work*/
}
document.querySelector('main').innerHTML = html;
Hope this helps.