Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial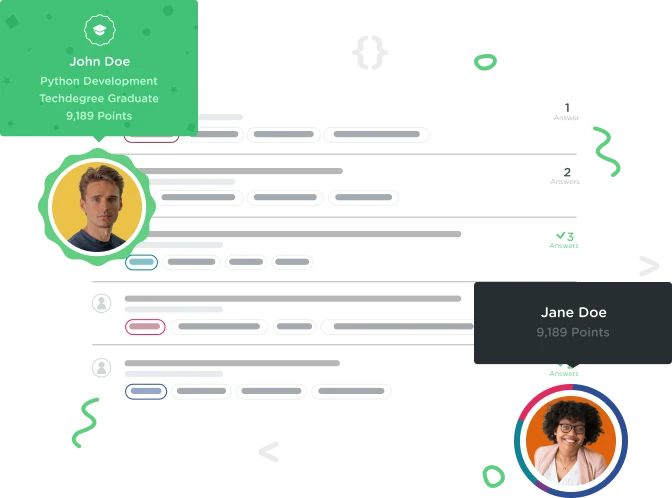

Haisem Jemal
631 PointsWhats the point of exceptions?
I don't really understand why you would have to use exceptions in java. It seems a little unnecessary. Can someone give me an example of when you would use it?
2 Answers

andren
28,558 PointsThe point of exceptions is essentially to communicate to the thing that called you that for one reason or another you can't really perform the action you were asked to do.
Take the Integer.parseInt()
method for example. That is a method that takes in a string and parses it as an int. Now what exactly do you think happens if the string that it is passed in does not actually contain a valid number for it to convert into an int?
Well the answer is that it throws an exception. And the reason for that is that there isn't really any alternative action to do. It can't return a number since there isn't one, and while it could in theory return a value like null
to indicate that it didn't find anything, doing that wouldn't really tell whatever called it anything about what error actually occurred.
On the receiving end since you can set up different catch blocks to deal with different exception types, you can have code that only runs during certain errors, which is really useful.
Errors are an unavoidable part of programming. Especially since you will often be dealing with things like user input, web connections, file reads / writes and other stuff which involve things that are outside of your control. It doesn't make sense to have the program crash whenever any error occurs, but it also doesn't make sense to just ignore them. So having an organized way of notifying that an error occurred, which allows for organized handling of those errors is essential. And that's what the exception systems allows developers to do.
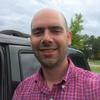
Brandon Adams
10,325 PointsIts so that when something happens in your program that should not happen, instead of the program crashing and locking up the computer, you can have the program give an error message and keep running.
For instance, someone puts numbers in their name and should only have letters, and the program does not know how to handle numbers, it can give an error message instead of crashing.
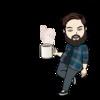
Philip Schultz
11,437 PointsHello Brandon, Why would you use this method instead of a while loop and an if statement that contains an error message? what is the advantage of Try throw and catch. For your name example, why not just do this:
do
{
System.out.println("Please enter your name: ");
name = ScannerObject.nextln();
if(!(name.matches("[a-zA-Z]+"))
{
System.out.println("Sorry, the name can only consist of alphabetical characters... please try again");
}
}while(!(name.matches("[a-zA-Z]+"))// this while loop