Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial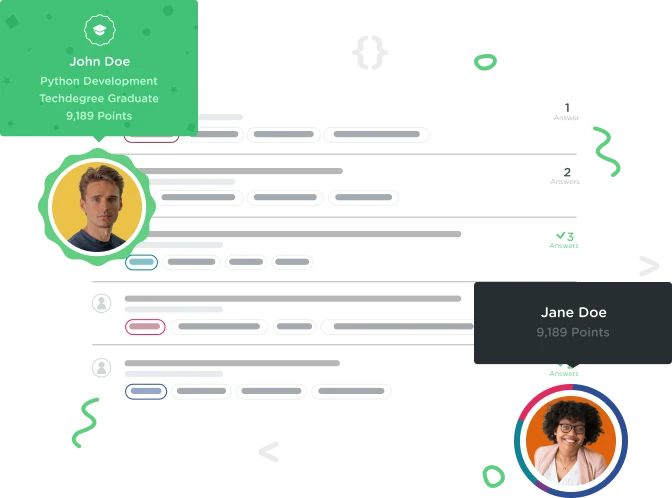
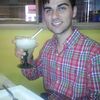
Nicholas Lee
12,474 PointsWhat's the point of parameters in a function?
In javascript, Why do we have to list parameters? I know it is necessary to list the arguments out for the function to work but does it serve another important purpose?
Perhaps so you can easily identify what is inside a function for debugging when reviewing code?
Thank you.
2 Answers
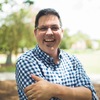
Dave McFarland
Treehouse TeacherActually, you don't have to list parameters in the function. Every JavaScript function receives it's own arguments
variable. This is an array containing all of the arguments passed to the function. You can access each argument using array notation:
function add() {
var results = 0;
for (var i = 0; i < arguments.length; i++) {
results += arguments[i];
}
return results;
}
add(1,2,3); // returns 6

Dino Paškvan
Courses Plus Student 44,108 PointsI'm not entirely sure I understand your question fully, but let me try to explain.
When defining a function, you must name the parameters to be able to refer to them inside the function in a readable manner.
function addTwoNumbers(a, b) {
return a + b;
}
While this function could be written like this:
function addTwoNumbers() {
return arguments[0] + arguments[1];
}
It's hardly readable, and anyone using your function wouldn't be able to tell how many parameters your function expects and what they should be.
Consider a slightly more complex scenario:
function greetMe(yourName, yourJob) {
return "Hello, " + yourName + ". I've heard you are a " + yourJob + ".";
}
If you were to use the arguments
Array-like object here, the code would be much harder to read.
Unnamed function parameters become a huge problem as your function grows more complex, and you do more things with them. If your function accepted 10 parameters, it would be extremely hard to track the position of each parameter to figure out what that parameter is.
Functions in all programming languages are modelled after mathematical functions, which turn a number of inputs (parameters) into an output (return value).
Bottom line, if you don't name your function parameters, other people (and even yourself) won't know how many of them there are and what they represent, and you'll have to resort to using the arguments
Array-like object to reference them inside the function, and actually do things with them.
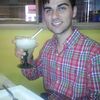
Nicholas Lee
12,474 PointsThis is what I was looking for. Makes more sense now in terms of its readability. thanks.
Nicholas Lee
12,474 PointsNicholas Lee
12,474 Pointsokay!