Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial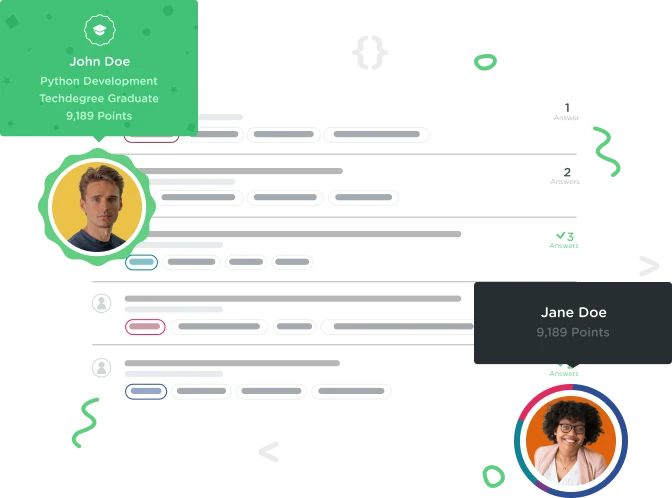
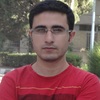
jabbar mehdipour
Courses Plus Student 604 Pointswhat's the problem with this code ? Should in the_list all non-integer values to be removed.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
index = 0
while True:
if type(the_list[index]) != int:
del the_list[index]
index = index + 1
if index >= len(the_list):
break
2 Answers

nightingale
2,056 PointsRemember when you delete and index it shift everything over so what you should try is:
if type(the_list[index]) != int: del the_list[index] else: index = index + 1
So it only increment when an int is found. For example slot 0 will get deleted and when you do that slot 1 will become 0 and so forth. So when it delete False the list then moves to the False position and since you have it to increment the list gets skipped.

Unsubscribed User
3,273 PointsWhile your method will work with the afore mentioned tweaks I think you would be better served by a for loop here since it would eliminate the need for your index = index + 1.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]] output_list = []
for value in the_list: if type(value) != int: output_list.append(value)
This will be a bit faster for a short list like this and will make your code a bit nicer to look at.
If you really want to keep your code style then one more simple change to make (in addition to what nighingale already told you) would be to replace index = index + 1 with index += 1. It does exactly the same thing but is a bit shorter, easier to look at, and quicker to type.
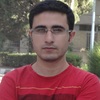
jabbar mehdipour
Courses Plus Student 604 Pointsaha , tanx . Finally, I wrote this code:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
index = 0
while index < len(the_list):
if type(the_list[index]) != int:
del the_list[index]
index -=1
index += 1
print(the_list)
And another question: Is there a for loop like C++ language in Python? like this :
for(int counter = 0 ; counter < sampleValue ; counter++){
//code...
}

Unsubscribed User
3,273 PointsThat looks kind of like a list comprehension...
I will admit I haven't done any work with C++ since I was in highschool (many many many years ago) and I didn't really get programming at all then so I can't really say that this is what you are asking for.
If I was you I'd look up some tutorials on list comprehension as I think that is what you are looking for.
Actually I'm surprised that the treehouse python track doesn't include a section on list comprehensions. What's up with that Mr. Love?
jabbar mehdipour
Courses Plus Student 604 Pointsjabbar mehdipour
Courses Plus Student 604 PointsRight. Thank you so much for your response.