Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial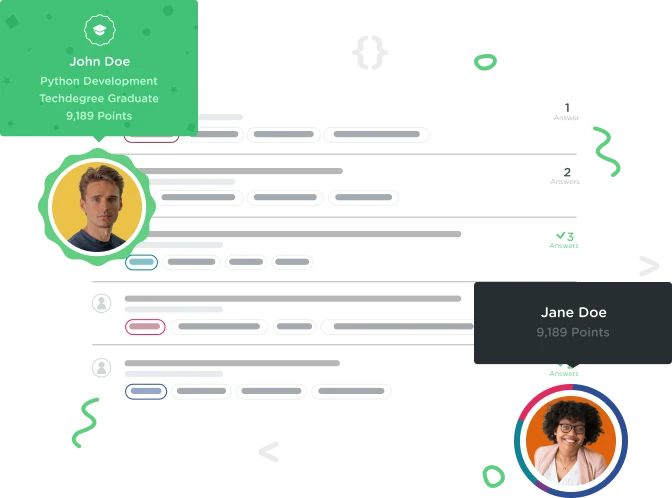
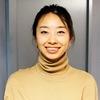
Flore W
4,744 PointsWhat's the role of axis = 0?
In the video, we are trying to append an array called "fake_log" to an existing two-dimensional array called "study_minutes"
study_minutes = np.append(study_minutes, [fake_log], axis=0)
Does axis=0 refer to the order in which the new array should be? Why in that case if I in put axis=1, I get a ValueError saying "all the input array dimensions except for the concatenation axis must match exactly"?
1 Answer
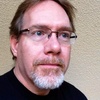
Chris Freeman
Treehouse Moderator 68,441 PointsIn the docs for numpy.append, the axis
refers to "The axis along which values are appended. If axis is not given, both arr and values are flattened before use."
The shape
method returns the number of array items along each axis! So appending along a given axes, increases the number of items along that axis.
The appended item must have the same number of dimensions. This means that you sometimes have to wrap the items to be appended by brackets [ ] to get the correct number dimensions
# A 1-dimensional array only has axis 0.
>>> np.array([1, 2, 3, 4, 5])
array([1, 2, 3, 4, 5])
>>> np.array([1, 2, 3, 4, 5]).shape
(5,)
# It can only be appended to on axis-0
>>> np.append(np.array([1, 2, 3, 4, 5]), [0], axis=0)
array([1, 2, 3, 4, 5, 0])
# Trying to append on axis-1 raises error
>>> np.append(np.array([1, 2, 3, 4, 5]), [0], axis=1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/chris/.virtualenvs/aoc/lib/python3.6/site-packages/numpy/lib/function_base.py", line 4528, in append
return concatenate((arr, values), axis=axis)
numpy.core._internal.AxisError: axis 1 is out of bounds for array of dimension 1
# A 2-d array with two axes
# axis-0 has length 2, and aixis-1 has length 5
>>> np.array([[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]])
array([[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10]])
>>> np.array([[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]]).shape
(2, 5)
# A 2-d array can be appended on either axis, **if** the appended array
# has the correct number of dimensions (In this case 2) and the correct shape
# The appended array must be the shape of the other dimension
# 2-d array appending a 1 by 5 array
>>> np.append(np.array([[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]]), [[91, 92, 93, 94, 95]], axis=0)
array([[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10],
[91, 92, 93, 94, 95]])
# here is the array that was amended
>>> np.array([[91, 92, 93, 94, 95]])
array([[91, 92, 93, 94, 95]])
# 2-d array appending a 2 by 1 array
>>> np.append(np.array([[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]]), [[96], [97]], axis=1)
array([[ 1, 2, 3, 4, 5, 96],
[ 6, 7, 8, 9, 10, 97]])
# here is the array that was amended
>>> np.array([[96], [97]])
array([[96],
[97]])
# A 3-d array can be appended one of three axis, **if** the appended array
# has the correct number of dimensions (in this case 3) and the correct shape
# The appended array must be the shape of the other two dimensions
# A 3-d array with three axes:
>>> np.array([[[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]],
... [[11, 12, 13, 14, 15], [16, 17, 18, 19, 20]],
... [[21, 22, 23, 24, 25], [26, 27, 28, 29, 30]]])
array([[[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10]],
[[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]],
[[21, 22, 23, 24, 25],
[26, 27, 28, 29, 30]]])
# This 3-d array has shape 3, 2, and 5 along axis-0, axis-1, and axis-2
>>> np.array([[[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]],
... [[11, 12, 13, 14, 15], [16, 17, 18, 19, 20]],
... [[21, 22, 23, 24, 25], [26, 27, 28, 29, 30]]]).shape
(3, 2, 5)
# The appended array must the *shape* of the other two dimensions
# 3-d array appended on axis-0 appending a 2 by 5 array:
>>> np.append(np.array([[[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]],
... [[11, 12, 13, 14, 15], [16, 17, 18, 19, 20]],
... [[21, 22, 23, 24, 25], [26, 27, 28, 29, 30]]]),
... [[[51, 52, 53, 54, 55], [56, 57, 58, 59, 60]]], axis=0)
array([[[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10]],
[[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]],
[[21, 22, 23, 24, 25],
[26, 27, 28, 29, 30]],
[[51, 52, 53, 54, 55],
[56, 57, 58, 59, 60]]])
# here is the array that was amended
>>> np.array([[[51, 52, 53, 54, 55], [56, 57, 58, 59, 60]]])
array([[[51, 52, 53, 54, 55],
[56, 57, 58, 59, 60]]])
# 3-d array appended on axis-1 appending a 3 by 5 array:
>>> np.append(np.array([[[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]],
... [[11, 12, 13, 14, 15], [16, 17, 18, 19, 20]],
... [[21, 22, 23, 24, 25], [26, 27, 28, 29, 30]]]),
... [[[61, 62, 63, 64, 65]], [[66, 67, 68, 69, 70]], [[71, 72, 73, 74, 75]]], axis=1)
array([[[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10],
[61, 62, 63, 64, 65]],
[[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20],
[66, 67, 68, 69, 70]],
[[21, 22, 23, 24, 25],
[26, 27, 28, 29, 30],
[71, 72, 73, 74, 75]]])
# here is array that was amended
>>> np.array([[[61, 62, 63, 64, 65]], [[66, 67, 68, 69, 70]], [[71, 72, 73, 74, 75]]])
array([[[61, 62, 63, 64, 65]],
[[66, 67, 68, 69, 70]],
[[71, 72, 73, 74, 75]]])
# 3-d array appended on axis-2 appending a 3 by 2 array:
>>> np.append(np.array([[[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]],
... [[11, 12, 13, 14, 15], [16, 17, 18, 19, 20]],
... [[21, 22, 23, 24, 25], [26, 27, 28, 29, 30]]]),
... [[[81], [82]], [[91], [92]], [[101], [102]]], axis=2)
array([[[ 1, 2, 3, 4, 5, 81],
[ 6, 7, 8, 9, 10, 82]],
[[ 11, 12, 13, 14, 15, 91],
[ 16, 17, 18, 19, 20, 92]],
[[ 21, 22, 23, 24, 25, 101],
[ 26, 27, 28, 29, 30, 102]]])
# here is array that was amended
>>> np.array([[[81], [82]], [[91], [92]], [[101], [102]]])
array([[[ 81],
[ 82]],
[[ 91],
[ 92]],
[[101],
[102]]])
Post back if you need more help. Good luck!!
Than Win Hline
1,679 PointsThan Win Hline
1,679 PointsI was struggling with axis. your explanation is really helpful.