Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial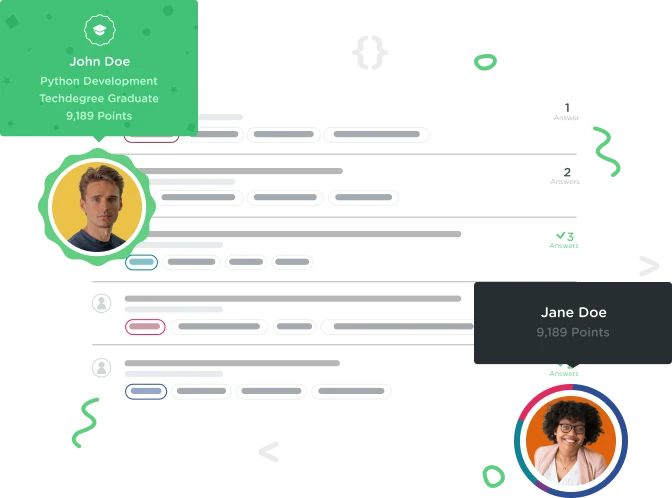

Vladislav Kobyakov
9,439 PointsWhat's the solution?
I was trying to make the init method as in the example from the previous video but still didn't get a few things. I compared the non-optional stored properties such as "title" and "author" to "nil" value as shown in the example before, but I do not really understand why do we compare a non-optional value to nil if it is never equal to nil since we know for sure that they exist?
Anyway, I couldn't really get how to make the init method at all in this exercise because an init method shouldn't return anything (unless it's "init?" and returns nil) like in this example. The code that I've written compiles but still doesn't pass the criteria.
I have feeling that the code that I've written is semantically completely wrong. Can someone please help me with it?
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]){
self.title = dict["title"]!
if self.title == nil{
return nil
}
self.author = dict["author"]!
if self.author == nil{
return nil
}
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
}
1 Answer

Heath Robertson
3,158 PointsHey. Here's my solution:
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]){
guard let title = dict["title"], let author = dict["author"] else {
return nil
}
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
}
I think you are supposed to use a guard statement, because you can initialise title and author first, because they will never be nil, then continue with the other parameters, checking if they are nil or not. The challenge probably wants you to use guard instead of if let, even though both work. In this case though, using guard is much cleaner. Hope that helped and made sense... :)
Vladislav Kobyakov
9,439 PointsVladislav Kobyakov
9,439 PointsThis makes sense, thank you! :)