Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial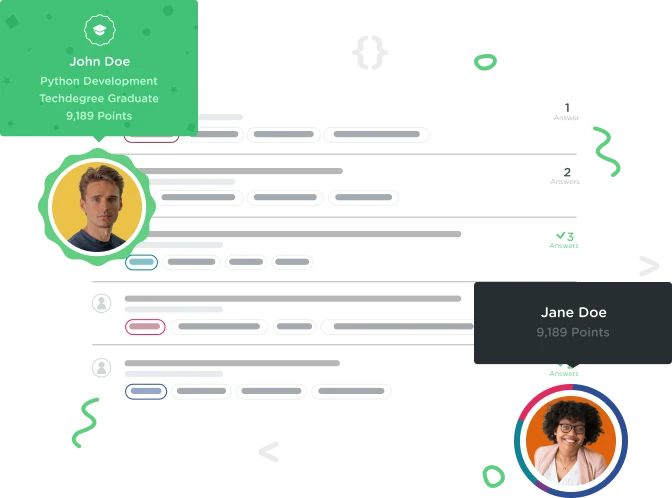

Raul Chiru
Courses Plus Student 2,776 Pointswhat's wrong?
Write the code inside this mimic_array_sum() function. That code should add up all individual numbers in the array and return the sum. You’ll need to use a foreach command to loop through the argument array, a working variable to keep the running total, and a return command to send the sum back to the main code.
<?php
function mimic_array_sum($array) {
foreach($array as $element) {
$count = $palindromic_primes[0] + $palindromic_primes[1] + $palindromic_primes[2];
}
}
return mimic_array_sum($array);
$palindromic_primes = array(11, 757, 16361);
?>
2 Answers
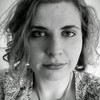
Grace Kelly
33,990 PointsHi Raul, first off you must place the return inside the mimic_array_sum function. Secondly inside your foreach loop you have the following:
$count = $palindromic_primes[0] + $palindromic_primes[1] + $palindromic_primes[2];
There's no way to know that each time a user inputs numbers, that they are always going to input 3...they might enter 2 or even a million, i would approach this in the following way:
<?php
function mimic_array_sum($array) {
$sum = 0;
foreach($array as $number) {
$sum += $number;
}
return $sum; //return the value of $sum
}
$palindromic_primes = array(11, 757, 16361);
?>
You declare a $sum variable outside the foreach loop and then add to it through each iteration of the loop so it goes like:
loop 1 - 0 + 11 = 11
loop 2 - 11 + 757 = 768
loop 3 - 768 + 16361 = 17129
This way the user can enter in as many numbers as they want and the code will still execute :)

Raul Chiru
Courses Plus Student 2,776 Pointsthank you!