Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial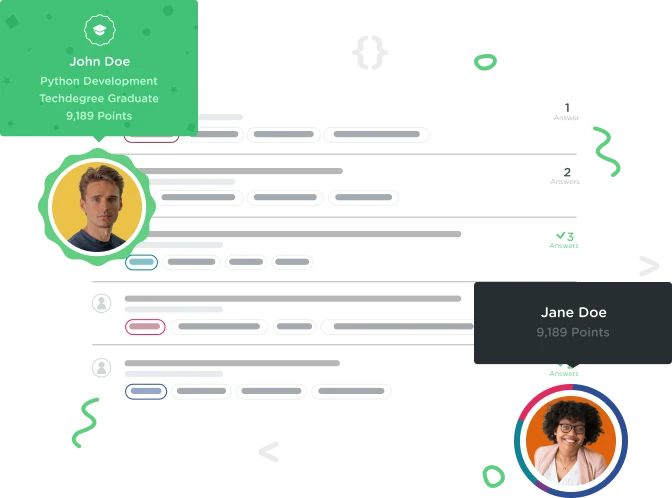
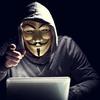
Pratham Mishra
Courses Plus Student 935 PointsWhat's wrong in this code?? Can i get help on this
package com.teamtreehouse; import java.util.Date;
public class Treet { private String mAuthor; private String mDescription; private Date mCreationDate;
public Treet(String author, String description, Date creationDate) { mAuthor = author; mDescription = description; mCreationDate = creationDate; }
@Override public String toString(){ return "Treet: " + "\" " + mDescription + "\"" + "\n -" + mAuthor ; }
@Override public int compareTo(Object obj){ Treet other = (Treet) obj; if(equals(other){ return 0; } int dtCmp = mCreationDate.compareTo(other.mCreationDate); if(dtCmp == 0){ return mDecription.compareTo(other.mDescription); } }
public String getAuthor() { return mAuthor; }
public String getDescription() { return mDescription; }
public Date getDate(){ return mCreationDate; }
public String[] getWords(){ return mDescription.toLowerCase().split("[^\w!]"); } }
//the other class
import com.teamtreehouse.Treet; import java.util.Date; import java.util.Arrays; public class Example{ public static void main(String args[]){ Treet treet = new Treet( "craigsdennis", "Want to be famous? Simply tweet about Java and use the " + "hashtag #treet. I'll use your tweet in a new " + "@treehouse course about Data Structures", new Date(1421849732000L) ); Treet secondTreet = new Treet( "journeytocode", "@treehouse make Java learning soooooo fun ! #treet", new Date(1421878767000L) ); System.out.printf("This is a new Treet: %s %n",treet); System.out.println("The words are:"); for(String word: treet.getWords()){ System.out.println(word); } Treet[] treets = {treet, secondTreet}; Arrays.sort(treets); for(Treet exampleTreet : treets){ System.out.println(exampleTreet); } } }
And it's giving error like:-
error: method does not override or implement a method from a supertype
@Override
2 Answers

Andrew Trachtman
3,680 Points@Override public int compareTo(Object obj){ Treet other = (Treet) obj; if(equals(other){ return 0; } int dtCmp = mCreationDate.compareTo(other.mCreationDate); if(dtCmp == 0){ return mDecription.compareTo(other.mDescription); } }
There are a few issues with the code you posted that I can see. First off, mDe >s< cription.compareTo(other.mDescription) You were missing the 's' there.
Secondly, I didn't see the implements Comparable up at the class level. This is required because if you never implement the interface, then the class assumes that you're trying to override the basic Java Object which doesn't have any such method called compareTo();. Because of that, there is nothing to override and the compiler gets upset. The @Override here without the "implements" is acting like any other @Override where it expects a superclass (Object here) to contain the method compareTo(). Once we have the "implements" at the top, the @Override becomes more of an indication that we're implementing something versus overriding something.
Lastly, you forgot to include the last return dateCmp; at the bottom. You need this because without it, there is no guarantee that the method you promised an int as a return value will ever get it.
public class Treet implements Comparable {
//...Other code here...
@Override
public int compareTo(Object obj){
Treet other = (Treet) obj;
if(equals(other)){
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if(dateCmp == 0){
//Any class can see private variables of other classes.
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
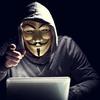
Pratham Mishra
Courses Plus Student 935 Pointsthanks Andrew. It solved my problem