Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial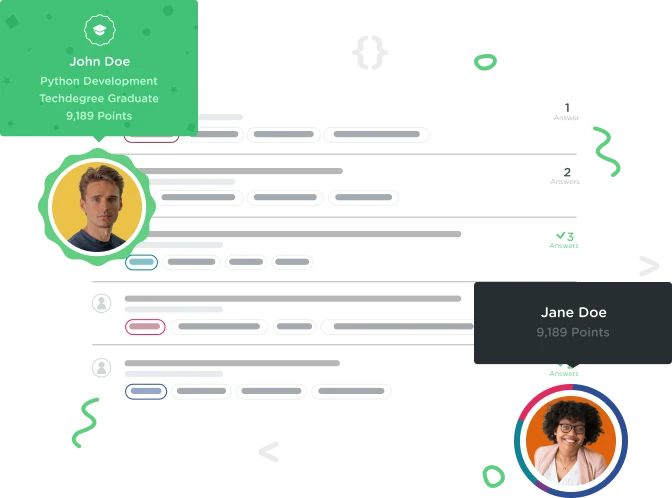

Matt Allen Dela Pena
4,561 PointsWhat's wrong with my code?
import random
make a list of words
words = [ 'apple', 'banana', 'orange', 'coconut', 'strawberry', 'lime', 'graprefruit', 'lemon', 'melon' ]
while True: start = input("Press enter/return to start, or type and enter Q to quit.") if start.lower() == 'q': break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw guessed letters, spaces, and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
# take guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("One letter at a time!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The secret word was {}.".format(secret_word))
break
else:
bad_guesses.append(guess)
else: print("You didn't guess it! The secret word was {}.".format(secret_word))
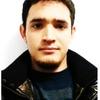
Anibal Marquina
9,523 PointsHi Matt, could you be a little bit more specific about your question to give you a hand?
To paste properly your code use the Markdown Cheatsheet.. you will find it at the bottom of the page with some exmaples
Cheers

Matt Allen Dela Pena
4,561 PointsApologies.
When I run the game, it generates infinite lines of the startup and then everything crashes.
import random
# make a list of words
words = [
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'graprefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
while True:
start = input("Press enter/return to start, or type and enter Q to quit.")
if start.lower() == 'q':
break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw guessed letters, spaces, and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
# take guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("One letter at a time!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The secret word was {}.".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it! The secret word was {}.".format(secret_word))
1 Answer
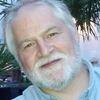
Jeff Muday
Treehouse Moderator 28,716 PointsYou are so close!
It is an indentation issue. From your comment "take a guess" down to the end needs to be indented by 4 spaces so it is part of your internal while loop.
Also you need to check the termination of the loop. Once you guess all the letters, you are going to have to check for that condition and exit the loop.
Matt Allen Dela Pena
4,561 PointsMatt Allen Dela Pena
4,561 Pointssorry that didn't paste right.