Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial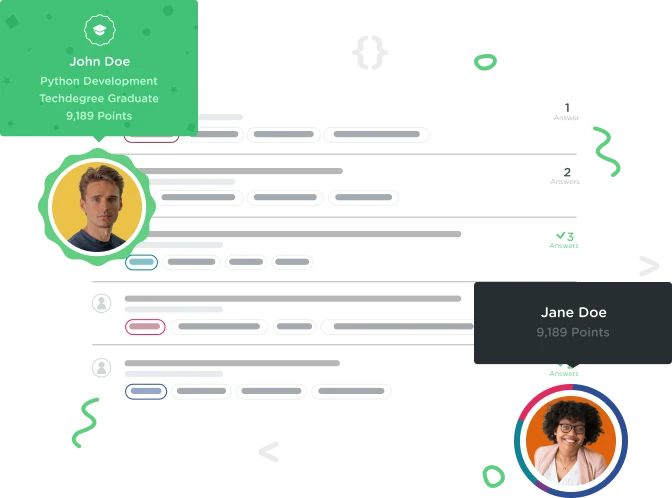
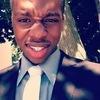
Jonathan Abdon
3,432 Pointswhat's wrong with my code?
I followed the instructions but still getting error that says try again. I can figure out why it is not letting me going to next task
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let d: String = (self.data?["someKey"])! else {
throw ParserError.emptyDictionary
}
let keys = self.data?.keys
guard (keys?.contains("someKey")) != nil else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
3 Answers
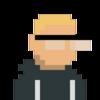
Everton Carneiro
15,994 PointsHello mate. Let's check your code:
func parse() throws {
guard let d: String = (self.data?["someKey"])! else {
throw ParserError.emptyDictionary
}
let keys = self.data?.keys
guard (keys?.contains("someKey")) != nil else {
throw ParserError.invalidKey
}
}
I'm not quite sure what you've tried to do, but this is not the way. In the first guard let statement you are not checking if the dictionary is empty, you are checking a key inside data. If after this you try to catch the erros with a do try catch block you'll notice that .emptyDictionary will be caught if your dictionary has "someKey" inside. Also if you pass an empty dictionary, your app will crash because you force unwrap and the compiler will find nil. Try like this:
func parse() throws {
//Check if the data dictionary is empty:
guard let data = data else {
throw ParserError.emptyDictionary
}
//Check if there's a key named "someKey" in the data dictionary:
guard let value = data["someKey"] else {
throw ParserError.invalidKey
}
}
Of course that in real world projects, I would pass the key as an argument of the function instead of hardcoding "someKey". But for the challenge it's enough. I hope that helps.
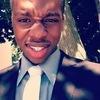
Jonathan Abdon
3,432 PointsThanks Everton Carneiro.

Brent Caswell
5,343 PointsThis answer didn't work for me. I needed to put a ?
after data
for it to work.
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let dictionary = data else {
throw ParserError.emptyDictionary
}
guard let value = data?["someKey"] else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)