Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial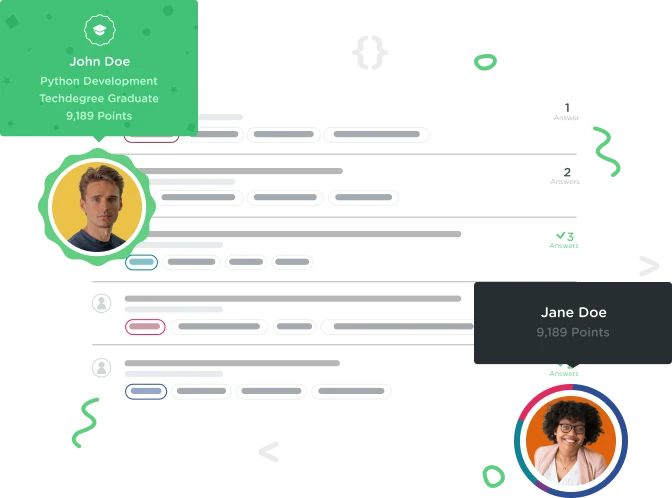

Benson Gathee
Courses Plus Student 1,280 PointsWhat's wrong with my code?
What's wrong with my code?
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
for(int i = 0; i < frogs.Length; i++)
{
double sum += frogs[i];
}
return sum/frogs.Length;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer
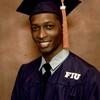
Dane Parchment
Treehouse Moderator 11,077 PointsSince you didn't show what the error was I am going to make an educated guess and say that it's because you are creating the variable: sum
inside the scope of the loop. This is going to create 3 specific problems.
Problem 1: Your variable is undefined upon creation, unless you instantiate it with a value. When you use the +=
operation, you are saying take the current value of this variable and add it to this other value, then set the variable equal to that value. The problem is your variable's value is null
. This is because like I said earlier you haven't actually given the variable sum
a default value. So it is like saying take null and add it to this value which in and of itself will lead to errors. I just don't remember off the top of my head if C# catches this error or not.
Problem 2: Your variable value is being re-created for each iteration of the loop. So even if the default value of your variable was say0
. You would be constantly setting the value of your variable to be:
0 + frogs[i]
- Iteration 1
0 + frogs[i]
- Iteration 2
0 + frogs[i]
- Iteration 3
Instead of the intended:
0 + frogs[i]
- Iteration 1
old_value + frogs[i]
- Iteration 2
old_value + frogs[i]
- Iteration 3
Where old_value
is the result of the previous addition.
Problem 3: You are referencing a variable that is created within a blocked scope, outside of that blocked scope. So basically the compiler doesn't recognize the variable sum
outside of that loop you created it in. Remember that variables created within a blocked scope: { } -- within curly braces
only exist within that scope, not outside of it.
With that it mind, how do you think you can solve your dilemma? Here is a tip, try creating the variable outside of the blocked scope, remember that you can still reference a variable from the global scope inside any other scope if you need to.
If you still need help let me know and I will provide the answer, I just want you to try it for yourself.