Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial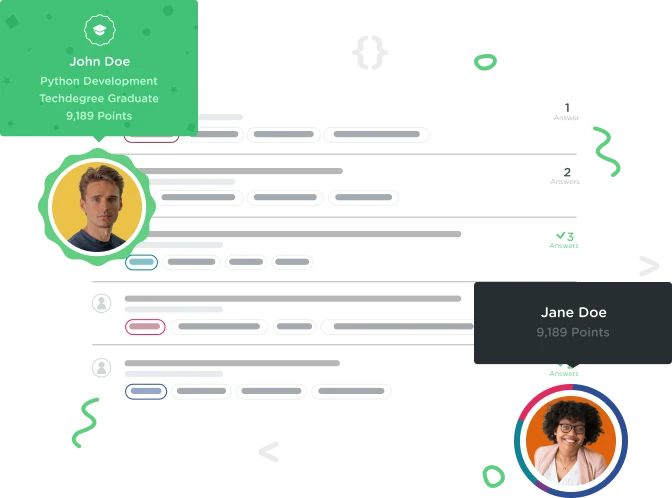
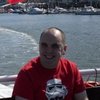
Sean Flanagan
33,235 PointsWhat's wrong with my code?
Hi. I've got a problem with this.
todo_list.rb:
require "./todo_item"
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
end
todo_list = TodoList.new("Groceries")
todo_item = TodoItem.new("Milk")
puts todo_item
todo_item.mark_complete!
puts todo_item
todo_item.mark_incomplete!
puts todo_item
todo_item.rb:
class TodoItem
attr_reader :name
def initialize(name)
@name = name
@complete = false
end
def to_s
if complete?
"[C] #{name}"
else
"[I] #{name}"
end
end
def complete
@complete
end
def mark_complete!
@complete = true
end
def mark_incomplete!
@complete = false
end
end
Error:
/home/treehouse/workspace/todo_item.rb:10:in `to_s': undefined method `complete?' for #<TodoItem:
0x0056255de57940 @name="Milk", @complete=false> (NoMethodError)
from todo_list.rb:14:in `puts'
from todo_list.rb:14:in `puts'
from todo_list.rb:14:in `<main>'
Does anyone know why this won't run please? Thanks. :-)
2 Answers
John Steer-Fowler
Courses Plus Student 11,734 PointsHi Sean,
Try entering this into todo_item.rb instead of your current version:
def to_s
if complete
"[C] #{name}"
else
"[I] #{name}"
end
end
Hope this fixes it

Oğulcan Girginc
24,848 PointsIn the todo_item.rb file, the if statement (10th line) calls the complete?
method. However, your method is named complete
(which is on 17th line) and doesn't have the question mark.
You can either delete the question mark as it was suggested or can add the question mark to your if statement.
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi John. I deleted the question mark and this is what I got:
Thanks for your help. :-)
John Steer-Fowler
Courses Plus Student 11,734 PointsJohn Steer-Fowler
Courses Plus Student 11,734 PointsNo problem.
It's fine to use question marks in method names, but you need to define the method with the question mark in it's name first.
Note that some built-in Ruby methods have question marks, but this is different to defining your own methods.
Glad I could help