Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial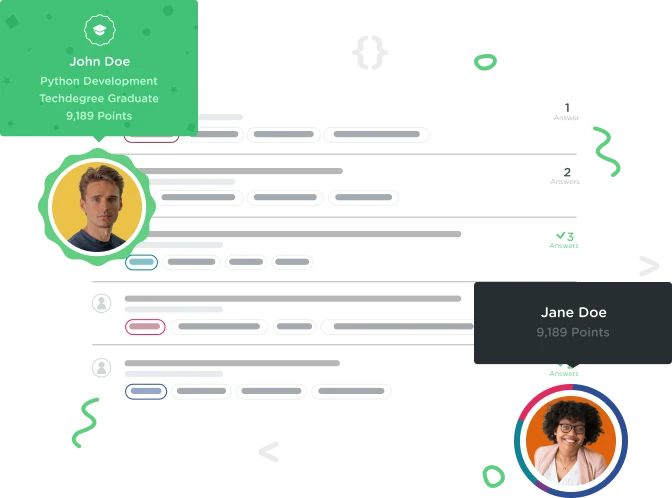
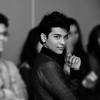
Vidit Shah
6,037 PointsWhats Wrong with my code?
Error: ./com/example/BlogPost.java:29: error: for-each not applicable to expression type for (BlogPost authorName : mPost) { ^ required: array or java.lang.Iterable found: String ./com/example/BlogPostFixture.java:9: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Alice", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:10: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Bob", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:11: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Charlie", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:12: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Alice", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:13: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Bob", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:14: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Charlie", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:15: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Dave", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:16: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Ephram", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:17: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Alice", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length ./com/example/BlogPostFixture.java:18: error: constructor BlogPost in class BlogPost cannot be applied to given types; new BlogPost("Alice", "TITLE", "BODY", "CATEGORY", new Date()) ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument lists differ in length Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 11 errors
showing in for Loop mPost
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private String mPost;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate,String post) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
mPost=post;
}
public Set<String> getAllAuthors() {
Set <String> set = new TreeSet<>();
for (BlogPost authorName : mPost) {
set.add(authorName.getAuthor());
}
return set;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
}
1 Answer
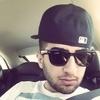
Vrezh Gulyan
11,161 PointsError: ./com/example/BlogPost.java:29: error: for-each not applicable to expression type for (BlogPost authorName : mPost) { ^ required: array or java.lang.Iterable found: String ./com/example/BlogPostFixture.java:9: error:
This first error in your logcat is saying that a for-each loop doesn't work with the type provided. It only works for an array or java.lang.Iterable , try using a regular for loop instead.
class BlogPost cannot be applied to given types; new BlogPost("Alice", "TITLE", "BODY", "CATEGORY", new Date()), ^ required: String,String,String,String,Date,String found: String,String,String,String,Date reason: actual and formal argument
This is the second error and it looks like it happens over and over in the logcat. This one is a simple error. The constructor that you declared for blogpost accepts:
public BlogPost(String author, String title, String body, String category, Date creationDate,String post) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
mPost=post;
}
String author, String title, String body, String category, Date creationDate,String post ^ the constructor has 6 parameters.
This is what you provided :
new BlogPost("Alice", "TITLE", "BODY", "CATEGORY", new Date())
You're only providing 5. You're missing the final string that supposed to be the post you're passing in.
Fix these errors and see what you get, I don't see any other problems in the logcat.
Vrezh Gulyan
11,161 PointsVrezh Gulyan
11,161 PointsI don't know if my answer helped you or not but if it did please mark it as the correct answer