Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial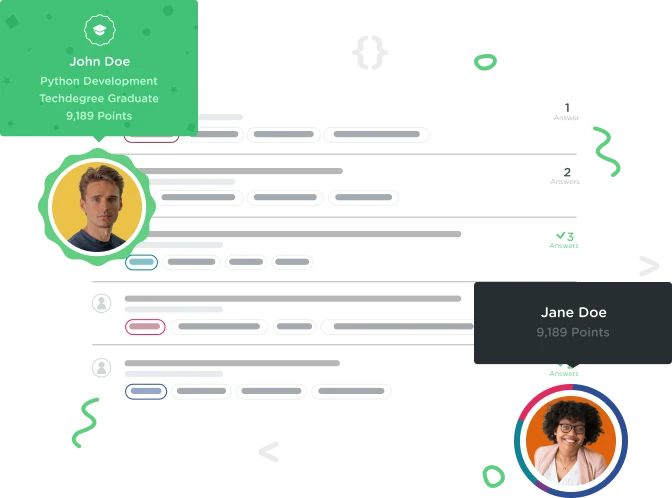
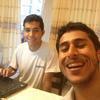
Wilfredo Casas
6,174 PointsWhat's wrong with my code?
Also, I'm not sure about the placeholder meaning
class Store():
open = 4
close = 8
def hours():
return "We're open from {} to {}.".format(open, close)
2 Answers
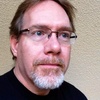
Chris Freeman
Treehouse Moderator 68,423 PointsYou are very close. You need to add the "self" reference for methods and attributes:
class Store():
open = 4
close = 8
def hours(self):
return "We're open from {} to {}.".format(self.open, self.close)
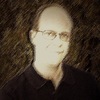
Jason Anders
Treehouse Moderator 145,858 PointsHey Wilfredo,
You're on the right track, but there are a couple things.
- When creating a Class, it does not take any parameters so shouldn't have () after it, you just need the colon.
Edited
- Now the method inside the Class needs a parameter (usual convention is
self
).
Answer edited to correct error
class Store:
open = 4
close = 8
def hours(self):
return "We're open from {} to {}.".format(self.open, self.close)
Hopefully this makes sense. Keep Coding!
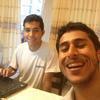
Wilfredo Casas
6,174 PointsThanks Jason, but is the self not necessary in the method of class? or does this version give me the same result?
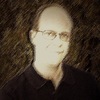
Jason Anders
Treehouse Moderator 145,858 PointsYou know, it's been a while since I've worked with Python, so this may be a question Chris Freeman can help with.
I did test the code I provided in the challenge, and it passed, but after reading Chris' answer, I'm actually not sure myself. Sorry.
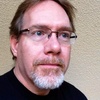
Chris Freeman
Treehouse Moderator 68,423 PointsWhile the parens after the class name are not required, it is common to use them as a visual cue that no inheritance (other than from the default object
) is defined.
Bound functions to classes (aka methods) require a first parameter to represent the bound instance or class. This can be named anything, but, again for visual cues, self
is used for instance methods and cls
is used for classmethods.
An alternative way to code the method hours()
would be to pass the default class attribute values for open
and close
in the parameter list. While this will pass the challenge it is likey not what you want.
class Store:
open = 4
close = 8
# add first parameter self and class attributes as defaults
def hours(self, open=open, close=close):
return "We're open from {} to {}.".format(open, close)
The default values for open
and close
are set at compile time, so "4" and "8" become the defaults even if open
and close
change:
# instantiate a new store
>>> s1 = Store()
# deg default hours
>>> s1.hours()
"We're open from 4 to 8."
# call hours with arguments
>>> s1.hours(1, 9)
"We're open from 1 to 9."
# set new hours
>>> s1.open = 9
>>> s1.close = 5
# verify hours
>>> s1.open
9
>>> s1.close
5
>>>
# check hours method. Still has predefined results
>>> s1.hours()
"We're open from 4 to 8."
By choosing to useself.open
and self.closeas arguments to
format()` the current value of these attributes will be used in the print.
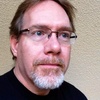
Chris Freeman
Treehouse Moderator 68,423 PointsJason, your posted code did not pass Task 2 when I tried it.
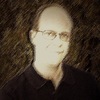
Jason Anders
Treehouse Moderator 145,858 PointsThank you very much Chris.
I edited my answer to correct the errors you pointed out. Again, thank-you for responding and for the detailed explanation (it's kind of coming back to me now). It was very helpful and very much appreciated.