Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial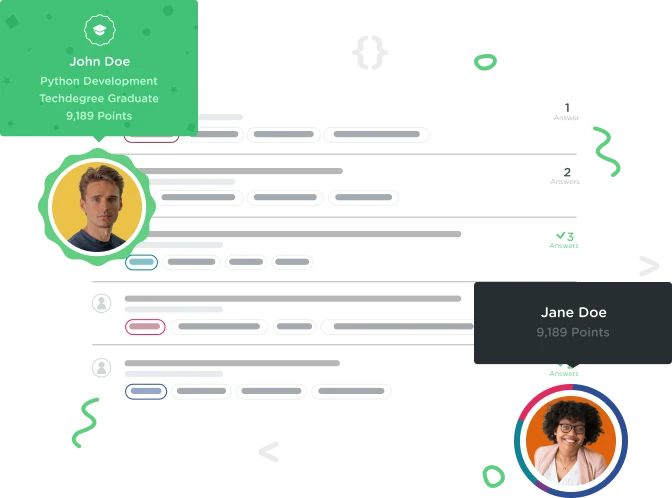
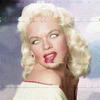
norman cole
2,756 Pointswhats wrong with my code thanks for the help
Now I want you to make a subclass of list. Name it Liar. Override the len method so that it always returns the wrong number of items in the list. For example, if a list has 5 members, the Liar class might say it has 8 or 2. You'll probably need super() for this.
import random
class Honest:
def __init__(self):
self.listofnums = []
def __len__(self):
return len(self.listofnums)
class Liar(Honest):
def __len__(self):
super().__len__(self)
if len(self.listofnums) == 2:
return random.randint(1, 10):
else:
return self.listofnums
1 Answer
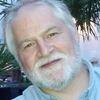
Jeff Muday
Treehouse Moderator 28,716 PointsThe challenge is a little simpler than you thought. You don't have to create an Honest class with encapsulated listofnums. The challenge just asks for a subclass of the Python 'list' type that returns a length that is always wrong. That can be accomplished by adding or subracting a non-zero integer to the value that is returned from the super class len(). The Liar class len function won't ever return the correct value -- but, the other list operations like append(), replace(), etc. as per the definition of a subclass will work as they should.
class Liar(list):
def __len__(self):
liar_len = super().__len__() + 1 # len() always lies because it is one more
return liar_len