Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial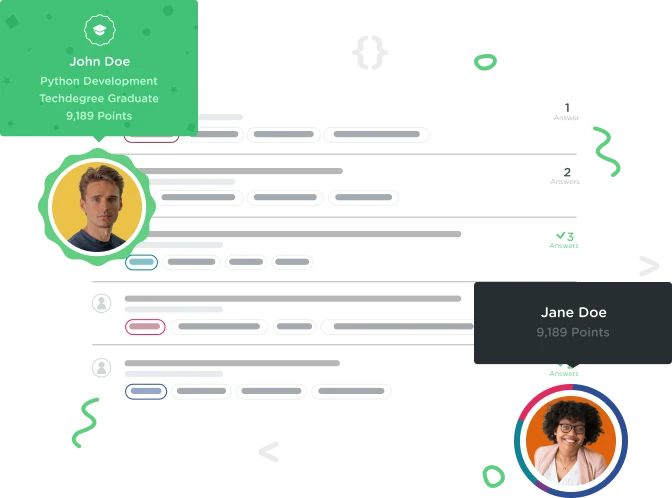

Aaron Coursolle
18,014 PointsWhat's wrong with my countdown?
Attempted this challenge and it took longer than I thought to get it working correctly. However the countdown (number of questions left) starts at 5 and doesn't update during the quiz. What did I do wrong?
//Countdown fails, displays only "5"; all other code works
//With the exception of question one,
//correct values are set to
//upper case
var correct1 = 12;
var correct2 = "JAVASCRIPT";
var correct3 = "CANON";
var correct4 = "BLUE";
var correct5 = "TORONTO"
//By default, all question values are set to false
var question1 = false;
var question2 = false;
var question3 = false;
var question4 = false;
var question5 = false;
//Countdown for questions left
var countdown = 5;
var questionsLeft = ' [' + countdown + ' questions left]';
//Intro
alert("Welcome To The Crown Quiz.");
alert("Please Answer The Following Questions.");
//Questions Section
//conditional statements will change correct (matching) answers to "true"
//first question is a parsed integer
var guess1 = parseInt(prompt("How many eggs in a dozen?" + questionsLeft));
countdown -= 1;
if (guess1 === correct1) {
question1 = true;
}
var guess2 = prompt("What language is this code written in?" + questionsLeft);
countdown -= 1;
if (guess2.toUpperCase() === correct2) {
question2 = true;
}
var guess3 = prompt("The Rebel Camera series are made by _____" + questionsLeft);
countdown -= 1;
if (guess3.toUpperCase() === correct3) {
question3 = true;
}
var guess4 = prompt("Why is the sky ______?" + questionsLeft);
countdown -= 1;
if (guess4.toUpperCase() === correct4) {
question4 = true;
}
//Question 5 doesn't get a "countdown -= 1;"
var guess5 = prompt("Type This: Toronto");
if (guess5.toUpperCase() === correct5) {
question5 = true;
}
//Testing purposes only
//to ensure conditional statements work
console.log(question1);
console.log(question2);
console.log(question3);
console.log(question4);
console.log(question5);
//Results Section
alert("Thank you for playing. Click ok for results.");
//declaring starting score
var score = 0;
//score is updated in this section
//of conditional statements and output
if (question1) {
score += 1;
document.write("<p>Question one was right!</p>");
} else {
document.write("<p>Question one was wrong. You should have said: " + correct1 + ".</p>");
}
if (question2) {
score += 1;
document.write("<p>Question two was right!</p>");
} else {
document.write("<p>Question two was wrong. You should have said: " + correct2 + ".</p>");
}
if (question3) {
score += 1;
document.write("<p>Question three was right!</p>");
} else {
document.write("<p>Question three was wrong. You should have said: " + correct3 + ".</p>");
}
if (question4) {
score += 1;
document.write("<p>Question four was right!</p>");
} else {
document.write("<p>Question four was wrong. You should have said: " + correct4 + ".</p>");
}
if (question5) {
score += 1;
document.write("<p>Question five was right!</p>");
} else {
document.write("<p>Question five was wrong. You should have said: " + correct5 + ".</p>");
}
//Ranking section
if (score === 0) {
var message = "<p>You didn't get any answers right. Better luck next time.</p>";
}
else {
//how range values are handled in JavaScript, the &&
//this is also a nested series of conditional statements
if (1 <= score && score <= 2) {
var crownColor = "bronze";
}
else if (3 <= score && score <= 4) {
var crownColor = "silver";
}
else if (score === 5){
var crownColor = "gold";
}
//output message if any of the nested series is true
var message = "<p>You got " + score;
message += " answers right, so you get a " + crownColor;
message += " crown. </p>";
}
//"message" variable used for both zero value and nested series
document.write(message);
1 Answer
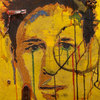
Eric Buchmann
15,080 PointsYou are actually decrementing countdown correctly, but the problem is in the the prompt. You are outputting the question and then the questionsLeft variable. The questionsLeft variable never updates. You would have to call your questionsLeft = ' [' + countdown + ' questions left]'; code again after each question to update the questionsLeft variable or find some other way to put the new countdown number in the prompt.
Aaron Coursolle
18,014 PointsAaron Coursolle
18,014 PointsThank you, the code is finally finished.