Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial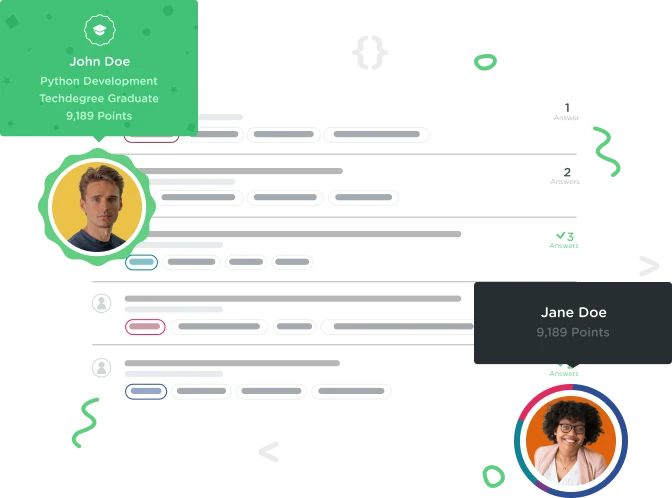

Evgeny Chuvelev
3,056 PointsWhat's wrong with my regexp to solve this issue?
I have error: Bummer! Didn't find the correct information for Pinckney Benton Stewart Pinchback.
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.match(r'((?P<last_name>[\w]+),? (?P<first_name>\w+),?):? (?P<score>\d+)', string, re.MULTILINE)
I check my regexp with finditer:
players = re.finditer(r'((?P<last_name>[\w]+),? (?P<first_name>\w+),?):? (?P<score>\d+)?', string, re.MULTILINE)
for player in players:
print '{last_name} {first_name} {score}'.format(**player.groupdict())
And I have output like this:
Love Kenneth 20
Chalkley Andrew 25
McFarland Dave 10
Kesten Joy 22
Stewart Pinchback None
Pinckney Benton 18
Where is a mistake?
Please help me, I really don't know how to solve this issue :(
3 Answers
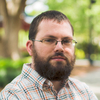
Kenneth Love
Treehouse Guest TeacherCheo R is right, you're not catching the right stuff for the first name. Not all first names are a single word. How would you capture word characters and spaces?

Evgeny Chuvelev
3,056 Pointsplayers = re.search(r'(?P<last_name>[\w\s]+),\s(?P<first_name>[\w\s]+): (?P<score>\d+)?', string, re.MULTILINE)
for player in players:
print '{last_name} {first_name} {score}'.format(**player.groupdict())
Result:
Love Kenneth 20
Chalkley Andrew 25
McFarland Dave 10
Kesten Joy 22
Stewart Pinchback Pinckney Benton 18
It's doesn't make sense :( Same error: Bummer! Didn't find the correct information for Pinckney Benton Stewart Pinchback. I don't get what I do wrong? :)
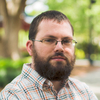
Kenneth Love
Treehouse Guest TeacherWhat parts don't make sense, Evgeny Chuvelev?

Evgeny Chuvelev
3,056 PointsIs regex in my last comment correct? I don't understand how make correct solution for this issue cause I don't know how correct answer should looks like to pass this task and don't get error "Bummer! Didn't find the correct information for Pinckney Benton Stewart Pinchback."
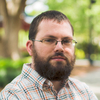
Kenneth Love
Treehouse Guest TeacherYour regex is almost correct. When you're using MULTILINE
, you want to be sure and mark the beginning of end of the lines in your pattern. That way the regex knows where and how to break the multi-line string to better match the pattern.

Evgeny Chuvelev
3,056 PointsThanks for help, Kenneth!
Cheo R
37,150 PointsCheo R
37,150 PointsIt looks like you're not getting the correct output, where the string is:
where
should be
and
should be