Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial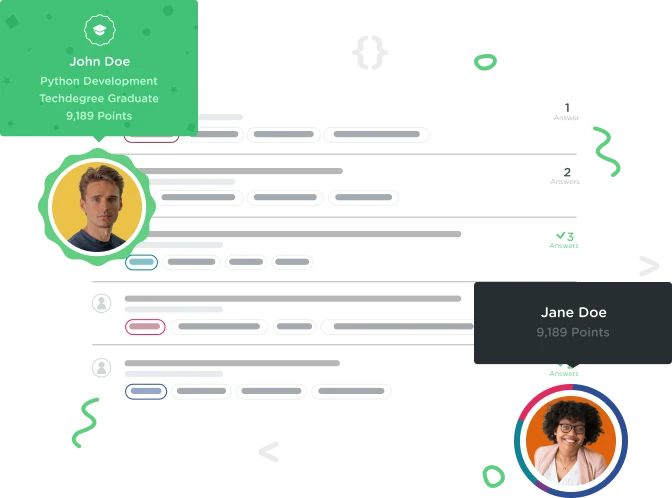

Aizah Sadiq
2,434 PointsWhat's wrong with the console?
Here's my code:
import random
class Die:
def __init__(self, sides=2, value=0):
if not sides >= 2:
raise ValueError("Must have at least 2 sides")
if not isinstance(sides, int):
raise ValueError("Must be a whole number")
self.value = value or random.randint = sides(1, sides)
I got this error:
treehouse:~/workspace/yatzy$ python
Python 3.6.4 (default, Nov 19 2019, 17:12:46)
[GCC 5.4.0 20160609] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from dice import Die
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/treehouse/workspace/yatzy/dice.py", line 11
self.value = value or random.randint = sides(1, sides)
^
SyntaxError: can't assign to operator
3 Answers
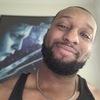
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsHi Aizah,
Two things. The first is, the error is being caused by this line of code:
self.value = value or random.randint = sides(1, sides)
Specifically, the issue is with the random.randint = sides(1, sides).
If you can explain what it is you’re trying to do with that particular part of the code, perhaps I can offer advice.
The second thing is this. You’ve set value to have a default value of 0, and so the second part of that statement (the part starting with or) will never be executed, because value will ALWAYS be defined. So right now, the code starting with or does nothing.
You should be able to simply delete it, and that error should go away. But if you’ve written that code to serve a purpose, like I said previously, let me know what it is you’re trying to do and perhaps I’ll be able to steer you in the right direction.

Aizah Sadiq
2,434 PointsThank you so much!!!

Aizah Sadiq
2,434 PointsI used that code to follow along with the video above and it serves the purpose of pulling out a random integer between 1 and the selected number of sides
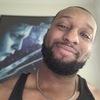
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsOK. First of all, my apologies. I said that the second expression in the or statement would never run because value already had a default value. I now realize that because 0 is a falsy value, 0 will be overridden if the second expression in the or statement evaluates as truthy.
That was new info for me (as I’ve tended to use if statements or ternary statements to check if an argument was passed in).
That said, the reason you’re receiving an error for the way you’ve currently written the or statement is because the second expression in the or statement attempts to store a tuple into random.randint as if it were a variable. But you’re already assigning the expression on the right side of the first = operator to self.value.
random.randint is a method (which is another name for a function that belongs to an object), and 1, and sides are meant to be passed into the method as arguments. If you go back and look at Kenneth’s code he’s not setting random.randint to be equal to (1, sides), he’s invoking random.randint like so: random.randint(1, sides)
Does this make sense? If not, let me know and I’ll try again.