Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial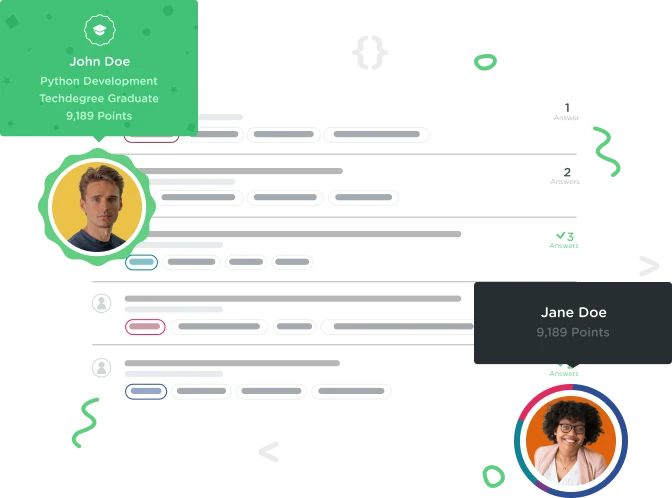

anilcherukuri
6,334 PointsWhat's wrong with the program?
Using the each method, iterate over the contact_list array. Assign each array item to the local variable contact in the block and print out the value of the name and phone_number keys.
contact_list = [{"name" => "Jason", "phone_number" => "123"}, {"name" => "Nick", "phone_number" => "456"} ]
contact_list.each do |item| contact = item contact.each_value do |value| puts value end end ​
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |item|
contact = item
contact.each_value do |value|
puts value
end
end
1 Answer

jonathanbello2
4,224 PointsThe loop is incorrect.
contact_list.each do |item| # This is going to do a full iteration
contact = item
contact.each_value do |value| # Here you are doing another within the first iteration.
puts value # you are only printing the value and not the key
end
end
What they are looking for is actually this:
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |item|
# Every iteration will take what item has and print key and value
puts item["name"]
puts item["phone_number"]
end
anilcherukuri
6,334 Pointsanilcherukuri
6,334 PointsJonathan,
Did you run my code? Even my code is giving the same output as yours.
Anil.