Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial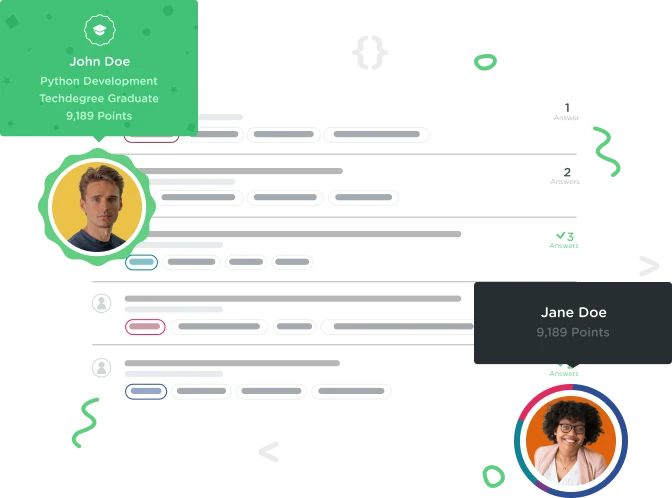

vivek d
iOS Development Techdegree Student 78 Pointswhats wrong with this?
can someone please help and explain
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data1 = data?.keys.contains("someKey") else {
throw ParserError.invalidKey
}
guard let data2 = data?.values.contains else {
throw ParserError.emptyDictionary
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
1 Answer
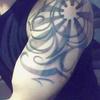
Paolo Scamardella
24,828 PointsSo it looks like your parse method implementation is not correct in the first place.
struct Parser {
var data: [String : String?]?
// This is how I would write it
func parse() throws {
// I would check if the dictionary is empty first
guard let result = self.data else {
throw ParserError.emptyDictionary
}
// Invalid key error will never be thrown.
// Why? Because contains method returns an optional true or false, and it will never be nil.
// The guard statement checks for nil, not false
// guard let data1 = data?.keys.contains("someKey") else {
// throw ParserError.invalidKey
// }
if self.data?.keys.contains("someKey") == false {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil] // no errors thrown
let data: [String: String?]? = nil // This will throw empty dictionary error
let data: [String: String?]? = ["someRandomKey": nil] // This will throw invalid key error because the key isn't someKey
let parser = Parser(data: data)
// usage of parser method
do {
try parser.parse()
catch ParserError.emptyDictionary {
print("Empty Dictionary")
// code to handle empty dictionary
} catch ParserError.invalidKey {
print("Invalid Key")
// code to handle invalid key/missing someKey key
}
All the parse method is doing is checking for if the dictionary is nil or the dictionary contains the wrong key. If the dictionary is nil, then it will throw an empty dictionary error. If the dictionary does not contain someKey key, then it will throw the invalid key error.
Hopefully this will make sense. If not, please be specific which part doesn't, so I can focus on that area for you.