Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial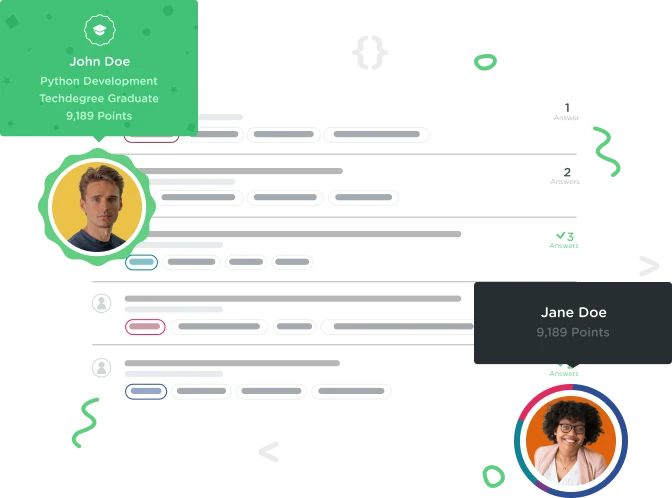

Michael Humenick
1,138 PointsWhat's wrong with this code?
//These are the 5 questions that the computer will ask the user.
var q1 = prompt('What is the first name of Mickle?');
var q2 = prompt('What is the second name of Mickle?');
var q3 = prompt('What is the first surname of Mickle?');
var q4 = prompt('What is the second surname of Mickle?');
var q5 = prompt('What is the full name of Mickle?');
//This is the variable that will determine how many correct answers you had at the end.
var correctAnswers = 0
//This line of code will check if the first question is correct.
if(q1 === Michael || michael || MICHAEL){
correctAnswers+=1
}else {
correctAnswers+=0
}
//This line of code will check if the second question is correct.
if(q2 === Terrance || terrance || TERRANCE){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This line of code will check if the third question is correct.
if(q3 === Humenick || humenick || HUMENICK){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This line of code will check if the fourth question is correct.
if(q4 === Cardona || cardona || CARDONA){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This line of code will check if the fifth question is correct.
if(q5 === Michael Terrance Humenick Cardona || michael terrance humenick cardona || MICHAEL TERRANCE HUMENICK CARDONA){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This will decide what alert to give the user, depending on the correct answers.
if(correctAnswers === 5){
alert('Congratulations! You got 5 out of 5! This means you have the ultimate badge... the gold badge!');
} else if(correctAnswers === 4 || 3){
alert('Congrats! you got ' + correctAnswers ' correct answers! This means you have obtained the silver badge.');
} else if(correctAnswers === 2 || 1){
alert('You did ok... I guess. You got ' + correctAnswers '. You got the bronze badge.'); }else if(correctAnswers === 0){
alert('You did horrible!!! You got zero correct answers. What is wrong with you?!?!?');
}
2 Answers
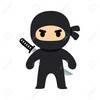
Dimitar Dimitrov
11,800 PointsYou forgot to concatenate the second part of you string here
alert('You did ok... I guess. You got ' + correctAnswers '. You got the bronze badge.'); !
It should look like this :
alert('You did ok... I guess. You got ' + correctAnswers + ' . You got the bronze badge.');
check your code you need to add "+ " to concatenate after the variable in other part of your code and delete the triple ``` at the very end of the code
When you open your browser console you can see at what line exactly is the error that will spare you some time
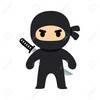
Dimitar Dimitrov
11,800 PointsYour conditional is kinda wrong if you want to test all 3 variants you should write it like this :
if(q1 === 'Michael' || q1==='michael' || q1=== 'MICHAEL')
Or you can just use :
if (q1.toLowerCase() === 'michael') and skip the other 2 variants.
Same with the others:
else if(correctAnswers === 4 || 3) this should looks like :
else if(correctAnswers === 4 || correctAnswers ====3)
Pay attention to the " " or ' ' when you are testing strings.

Michael Humenick
1,138 PointsI changed what you said. But it still doesn't work. I'm sure its my fault and I'm being an idiot. Its jus not asking the questions at the beginning!
//These are the 5 questions that the computer will ask the user.
var q1 = prompt('What is the first name of Mickle?');
var q2 = prompt('What is the second name of Mickle?');
var q3 = prompt('What is the first surname of Mickle?');
var q4 = prompt('What is the second surname of Mickle?');
var q5 = prompt('What is the full name of Mickle?');
//This is the variable that will determine how many correct answers you had at the end.
var correctAnswers = 0
//This line of code will check if the first question is correct.
if(q1 === 'Michael' || q1 === 'michael' || q1 === 'MICHAEL'){
correctAnswers+=1
}else {
correctAnswers+=0
}
//This line of code will check if the second question is correct.
if(q2 === 'Terrance' || q2 === 'terrance' || q2 === 'TERRANCE'){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This line of code will check if the third question is correct.
if(q3 === 'Humenick' || q3 === 'humenick' || q3 === 'HUMENICK'){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This line of code will check if the fourth question is correct.
if(q4 === 'Cardona' || q4 === 'cardona' || q5 === 'CARDONA'){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This line of code will check if the fifth question is correct.
if(q5 === 'Michael Terrance Humenick Cardona' || q5=== 'michael terrance humenick cardona' || q5=== 'MICHAEL TERRANCE HUMENICK CARDONA'){
correctAnswers+=1
} else {
correctAnswers+=0
}
//This will decide what alert to give the user, depending on the correct answers.
if(correctAnswers === 5){
alert('Congratulations! You got 5 out of 5! This means you have the ultimate badge... the gold badge!');
} else if(correctAnswers === 4 || correctAnswers === 3){
alert('Congrats! you got ' + correctAnswers ' correct answers! This means you have obtained the silver badge.');
} else if(correctAnswers === 2 || correctAnswers === 1){
alert('You did ok... I guess. You got ' + correctAnswers '. You got the bronze badge.');
}else if(correctAnswers === 0){
alert('You did horrible!!! You got zero correct answers. What is wrong with you?!?!?');
}```