Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial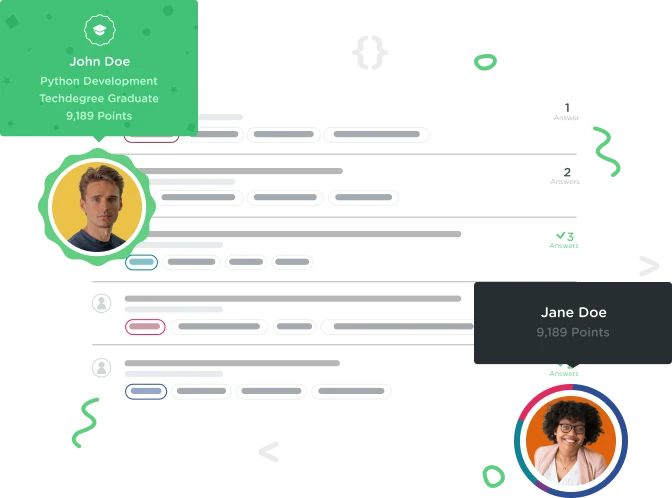

David Frenkel
14,548 PointsWhats wrong with this? It runs perfectly in Xcode!
Any ideas?
/**
For this code challenge, letβs define a math operation as a function that
carries out some work on two integers and returns an integer as well. An
example is the function below, `differenceBetweenNumbers`, which takes two
integers and calculates the difference between the numbers. After calculating,
it returns the difference.
*/
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation (operation: (first: Int, second: Int) -> (Int), firstInt: Int, secondInt: Int ) -> Int {
return operation(a: firstInt, b: secondInt)
}
edit the ask question button copied in the code mid editing this is the one I wrote and wouldn't compile:
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation (mathOperation: (a:Int, b:Int) -> (Int), int1: Int, int2: Int ) -> Int {
let something = mathOperation(a: int1, b: int2)
return something
}
print(mathOperation(differenceBetweenNumbers, int1: 6, int2: 5))
2 Answers
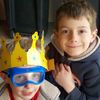
Chris Stromberg
Courses Plus Student 13,389 PointsI know my code was fine and no it was not the same as mine until you edited it. I was trying to tell you that your code was bad because you were trying to return a parameter named "a" and "b" that did not exist in your function signature.
I'm curious as to why you are naming the parameters of the function that is passed in as a parameter for "mathOperation"? I get it you can do it that way, but the question didn't ask for that.
Your code...
func mathOperation (operation: (first: Int, second: Int) -> (Int)
Why not just submit the code below that does not name the parameters of the function passed in to your function? In addition, the body of your function is suppose to just return the variables passed in, not assign them to a constant that is returned.
func mathOperation(operation:(Int,Int) -> (Int),a:Int,b:Int) -> Int
{
return operation(a,b)
}
Or if you want to stick with your naming conventions......
func mathOperation (mathOperation: (Int,Int) -> (Int), int1: Int, int2: Int ) -> Int {
return mathOperation(int1, int2)
}
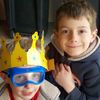
Chris Stromberg
Courses Plus Student 13,389 PointsYour code below will not compile in Xcode. You are passing two parameters into your return statement named "a" and "b", yet they are no where in your function signature.
func mathOperation (operation: (first: Int, second: Int) -> (Int), firstInt: Int, secondInt: Int ) -> Int {
return operation(a: firstInt, b: secondInt)
}
This will.
func mathOperation (operation: (first: Int, second: Int) -> (Int), firstInt: Int, secondInt: Int ) -> Int {
return operation(first: firstInt, second: secondInt)
}
Or you could do a lot less typing and just submit this.
func mathOperation(operation:(Int,Int) -> (Int),a:Int,b:Int) -> Int
{
return operation(a,b)
}
And call it.
mathOperationTwo(differenceBetweenNumbers, 5, 2)

David Frenkel
14,548 PointsYour code is fine, it's same is mine (check edit) but the challenge wont accept it.