Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial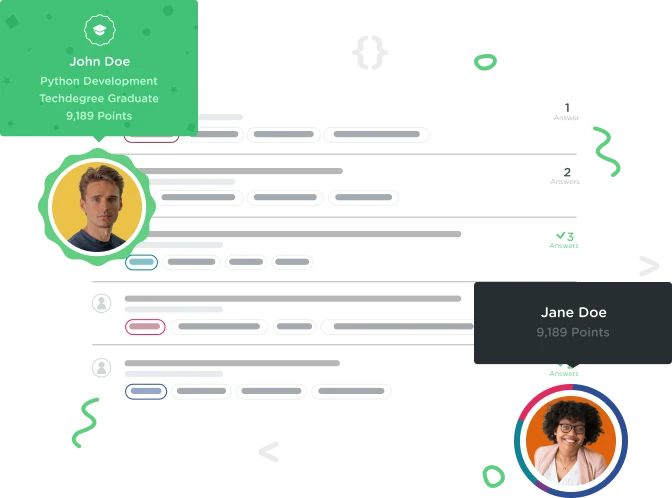

Till de Vries
3,837 PointsWhats wrong with this simple for...in loop
Hey guys,
why the following for...in loop produces:
Site shows:
0:[object Object]
1:[object Object]
Code is:
var lala = [
{name: "lala", size: "lala", weight: 88},
{name: "lala", size: "lala", weight: 88}
];
for (var prop in lala){
document.write('<p>' + prop + ":" + lala[prop] + '</p>');
}
Thank you in advance!!
2 Answers
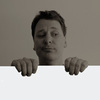
Sean T. Unwin
28,690 PointsSince the goal is to get the object's properties and values, where the object is inside an array, we will need to use two (2) loops. One to loop through the array and another to loop through the object.
Below is how I did it:
Note: I used console.log()
as I tested in Nodejs. You may substitue for document.write()
if you wish.
var lala = [
{name: "lala", size: "lala", weight: 88},
{name: "lala", size: "lala", weight: 88}
];
// Loop through the Array
for (var item in lala) {
// Output Array item
console.log('item[' + item + ']: ' + lala[item]);
// Loop through Object
for (var prop in lala[item]){
// Output Object property and value
console.log(' |- ' + prop + ": " + lala[item][prop]);
}
}
Output is as follows:
item[0]: [object Object]
|- name: lala
|- size: lala
|- weight: 88
item[1]: [object Object]
|- name: lala
|- size: lala
|- weight: 88
If you have any other questions feel free to ask. :)

YI LI
4,094 PointsThe lala
variable > var lala = [ {name: "lala", size: "lala", weight: 88}, {name: "lala", size: "lala", weight: 88} ];
indicates the two object are wrapped by array,
Since for...in...
statement loop through an array and return the index, the prop
in for (var prop in lala)
will be its index and lala[prop]
will be each object (however, MDN says "There is no guarantee that for...in
will return the indexes in any particular order ........" https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for...in)
That is, lala[0] -> {name: "lala", size: "lala", weight: 88}
, lala[1]-> {name: "lala", size: "lala", weight: 88}
if you want to get the properties separately, try
for(var ele =0; ele<lala.length; ele++){
for(prop in lala[ele]){
document.write(prop + ":" + lala[ele][prop]);
}
}
Hope it helps you.

Till de Vries
3,837 PointsYes it does YI LI - thx!

Iain Simmons
Treehouse Moderator 32,305 PointsHi YI LI, very good point about for...in
and arrays. I've added some code formatting to your answer to make it clearer.
Till de Vries
3,837 PointsTill de Vries
3,837 PointsI got it - thank you Sean !
mk37
10,271 Pointsmk37
10,271 Pointshey, i know this question is old now but i though i still give it a shot. i understand how the for-in loops works here, thank the explanation on that. but im still not understanding why:
lala[item]
produces '[object Object]' as output . it dont make sense to me, i can only guess somehow this is javascript counter measure or something