Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial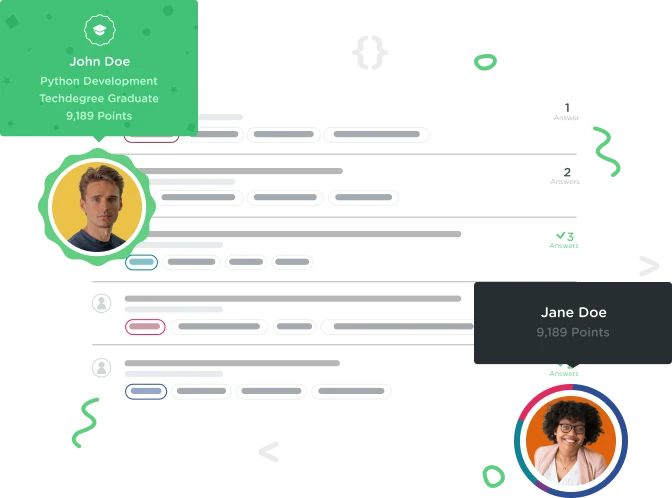

Nathan Yellon
5,452 PointsWhen adding a task the <label> is undefined and I can't get it to show the text that I enter in the add item input
var taskInput = document.getElementById("new-task"); //#new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //<ul #incomplete-tasks>
var completedTasksHolder = document.getElementById("completed-tasks"); //<ul #completed-tasks>
//New Task List Item
var createNewTaskElement = function (taskString) {
var listItem = document.createElement("li");
var checkBox = document.createElement("input");
var label = document.createElement("label");
var editInput = document.createElement("input");
var editButton = document.createElement("button");
var deleteButton = document.createElement("button");
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
var addTask = function(taskInput) {
console.log("Add Task");
//create new list item with the text from #new-task
var listItem = createNewTaskElement(taskInput.val);
//append list item to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
var editTask = function() {
console.log("Edit Task");
}
var deleteTask = function() {
console.log("Delete Task");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//remove parent list item from ul
ul.removeChild(listItem);
}
var taskCompleted = function() {
console.log("complete Task");
//append list item to completed tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
var taskIncomplete = function() {
console.log("incomplete Task");
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("bind list items");
//select list item's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i ++) {
//bind events to list items children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completeTasksHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i ++) {
//bind events to list items children (taskIncompleted)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
1 Answer
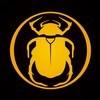
rydavim
18,814 PointsOkay! It seems like there were a couple things not working.
// In your addTask function, you don't need to pass the parameter because it is global.
// You also want to use the full word 'value' to get the value.
var addTask = function() { // removed parameter
var listItem = createNewTaskElement(taskInput.value); // changed val to value
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
// In your createNewTaskElement function, when setting the label text, it looks like innerText doesn't work.
// Try using textContent instead.
label.textContent = taskString; // changed innerText to textContent
That should do it, but let me know if those changes don't work for you or if you have any other questions. Happy coding! :)

Nathan Yellon
5,452 PointsThanks i got it!

tony stark
Courses Plus Student 1,584 Pointsyou seem to understand this. I can't figure out how to implement the teachers note on this video. Teachers note:
innerText and Firefox Mozilla didn't implement innerText but has implemented textContent. innerText can be used in older versions of Internet Explorer and all versions of Chrome and Safari. textContent can be used in Internet Explorer 9 onwards and all other browsers. Here's some cross-browser compatible code for the edit button:
if (typeof editButton.innerText === "undefined") {
editButton.textContent = "Edit";
} else {
editButton.innerText = "Edit";
}
rydavim
18,814 Pointsrydavim
18,814 PointsI've added some code formatting to your post. You can do this using the following markdown:
```language
your code here
```