Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial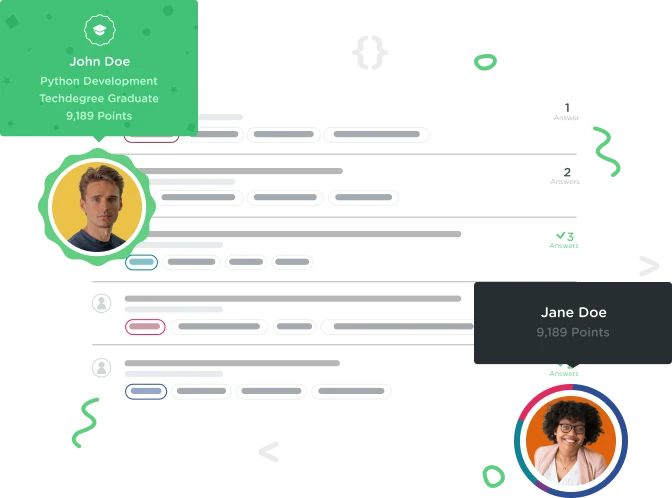
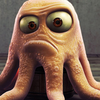
Laurence Foley
16,695 PointsWhen checking for localStorage support, why not just use '' if(localStorage){} '' ?
I dont get why you wouldn't just use something as simple as this to check for local storage support.
if (localStorage) {
// Local storage is supported
}
Even Mozilla's example seems unnecessary complex.
//Mozilla's example to detect local storage support and availability
function storageAvailable(type) {
try {
var storage = window[type],
x = '__storage_test__';
storage.setItem(x, x);
storage.removeItem(x);
return true;
}
catch(e) {
return false;
}
}
if (storageAvailable('localStorage')) {
// Yippee! We can use localStorage awesomeness
}
else {
// Too bad, no localStorage for us
}
3 Answers

Stijn de Witt
33 PointsI had to create a trial account just to provide this answer... but here goes:
I dont get why you wouldn't just use something as simple as this to check for local storage support.
if (localStorage) { // Local storage is supported }
The reason here is that browser vendors messed this up.
First of all, this feature detection will fail with an exception if the symbol localStorage
does not exist. Similar to how if (whatever) {}
will fail if window
does not have a property whatever
. You would have to test below window
explicitly, like this: if (window.localStorage)
.
However, some browsers will actually throw an exception if you 'touch' the localStorage object like this... This is why some people test with if ('localStorage' in window) {}
.
However, even this is not enough. The reason is that some browsers provide a dummy localStorage object even though they do not actually support it...
And finally there is Safari. Safari in private browsing mode gives you a functional local storage, but sets its quotum to zero. This is a very strange decision imho. I would have expected it to work like cookies: you can read and write them, but they will be wiped as soon as you close the browser session. This is how FF and Chrome implemented it, but alas Safari chose different.
And that is how we end up at this situation where we write a key into localStorage and then remove it again. We also catch any exceptions here.
apparently your suggestion is perfectly valid.
Trust me, it is not. Your code will break in the wild.
Why do we have to check for " 'localStorage' in window " and, after this is true, for " window['localStorage'] !== null ". Shouldn't be enough with the second condition, that includes the first one?
No. 'x' in object
tests whether the object has a property with name 'x'. It will return true, even if the value of that property is null
or undefined
:
var object = { x: undefined }
'x' in object // true
var object = { x: null }
'x' in object // true
var object = { y: 1 }
'x' in object // false
Mozilla's example seems unnecessary complex.
It is not. In fact it is about as simple as I could make it. I am the author of this part of the MDN and also the package storage-available on NPM.
For a more complete history of how we (the community) ended up with this specific solution, see this Github Gist: A brief history of detecting local storage
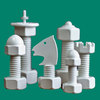
Steven Parker
231,275 PointsElsewhere on MDN I have seen this example:
if (!window.localStorage) {
So apparently your suggestion is perfectly valid.

ludopuig
Full Stack JavaScript Techdegree Student 6,436 PointsHi!
A question related to this one... about the return statement in line 30, which says:
return 'localStorage' in window && window['localStorage'] !== null
Why do we have to check for " 'localStorage' in window " and, after this is true, for " window['localStorage'] !== null ". Shouldn't be enough with the second condition, that includes the first one?
Tomasz Cysewski
2,736 PointsTomasz Cysewski
2,736 Pointstry catch is special syntax for checking if there is an error, so we all use it.