Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial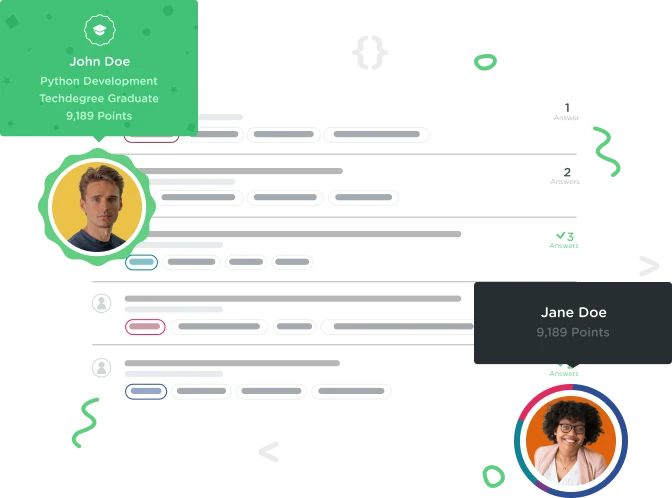

Aaron Bailey
iOS Development Techdegree Student 305 PointsWhen do Unary Operators and Operators become useful in coding and what is the purpose of them?
?
1 Answer
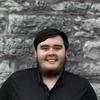
Michael Hulet
47,913 PointsUnary operators are operators that operate on a single operand. In other words, instead of going between 2 variables, it goes only before or after 1 variable. Off the top of my head, I can think of 3 unary operators in Swift: the logical not operator (!
before a boolean), the optional chaining operator (?
), and the forcible optional unwrapping operator (!
after an optional).
The logical not operator (!
before a boolean) inverts the boolean that it operates on. If its variable is true
, it returns false
, but if its variable is already false
, it returns true
. It's most frequently used in if
statements to check if something is false
. In practice, it looks like this:
var some = true
some = !some
dump(some) // some is now false
some = !some
dump(some) // some is now true again
The optional chaining operator (?
) is useful when you want to access a nested optional property, but it doesn't really matter if any optional in the chain fails. If its optional exists, it will unwrap it. If its optional is nil
, it's no big deal. Your program won't crash due to finding a nil optional. It'll just break the chain and the property/method you were trying to access will not be, and the rest of the line will be skipped. It's most often useful for assignment. This is what it looks like in code:
struct Something{
var sub: Other? = nil // Don't confuse the optional chaining operator with an optional type. In an optional type, the question mark (?) is part of the type signature
}
struct Other{
var number: Int? = nil
}
var instance: Something? = nil
instance?.sub?.number = 4 // Assignment will not happen because instance is nil
instance = Something()
instance?.sub?.number = 4 // Assignment will not happen because sub is nil
instance?.sub = Other() // Assignment will succeed because instance is not nil
instance?.sub?.number = 4 // Assignment will succeed because both instance and sub are not nil
The forcible optional unwrapping operator (!
after an optional) is like the optional chaining operator, but it's generally considered something that's bad to use and you should avoid because your app **will* crash if its optional is nil
*. However, if its optional is not nil
, it will unwrap it inline. In code, it looks like this:
var some: Int? = 1
dump(some!) // Prints 1
some = nil
dump(some!) // Your app will crash
Previous versions of Swift had 2 other operators for incrementing and decrementing a number, but those were removed in Swift 3 because they were fairly obscure and their semantics were hard to remember. They could go either before or after the variable they operated on. If they were before their variable, they would increment or decrement their variable and return its new value, but if they went after their variable, it would return their variable's current value and then increment or decrement its value. ++
would increment a variable and --
would decrement it. In code, they looked like this:
var some = 1
dump(++some) // Prints 2. some is now equal to 2
dump(--some) // Prints 1. some is now equal to 1 again
dump(some++) // Prints 1, but some is now equal to 2
dump(some--) // Prints 2, but some is now equal to 1 again
One of the cool things about Swift is that it allows you to overload existing operators or define your own new ones. Because of this, unary operators in Swift can be whatever you want to make. If you have a good idea for one, feel free to implement it! Ultimately, the key takeaway is this: Unary operators are operators that operate on only one operand instead of 2