Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial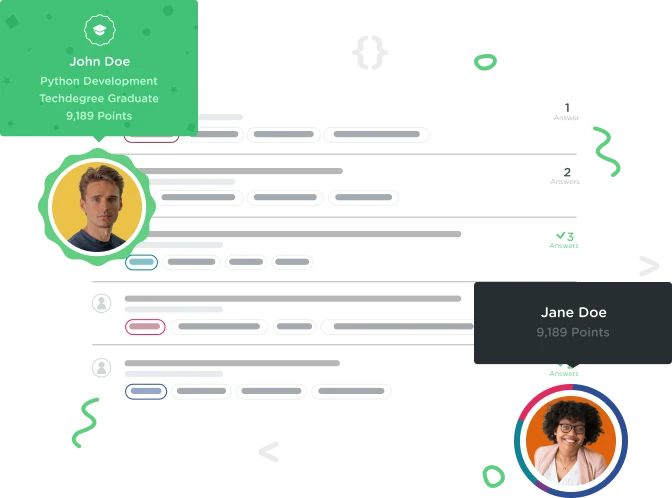

Luca Di Pinto
8,051 PointsWhen do we need to use for-in loop?
Hi guys, could you explain me better which is the difference between a normal loop and a for-in loop? It's difficult to understand which are the situations when to use one instead of the other one. Thanks for the clarification! Luca
2 Answers
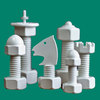
Steven Parker
230,274 PointsIdan's right about for..in being handy for objects and properties. But it's also good for arrays.
For example, his array loop example that uses .length can be re-written this way:
var squares = [1, 4, 9, 16, 25, 36];
for ( var i in squares ) {
console.log(squares[i]);
}

Luca Di Pinto
8,051 PointsSo I think it's always useful to use for... in loops, ins't it?
Idan Melamed
16,285 PointsIdan Melamed
16,285 PointsHi Luca, I'm just taking this course as you, so I'm not sure my answer will be 100% correct.
A for loop looks like this:
In this example the loop will run exactly 10 times. So regular loops are great for when you know exactly how many times you need to run the through the loop. It is really good for going through arrays, because the first item in an array is array[0], the second item is array[1] and so on... And you can always use the 'length' property of an array to serve as your condition. Like this:
The for...in loop goes through the properties of objects. For example, if you want to log all the values of:
You can do it with: