Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial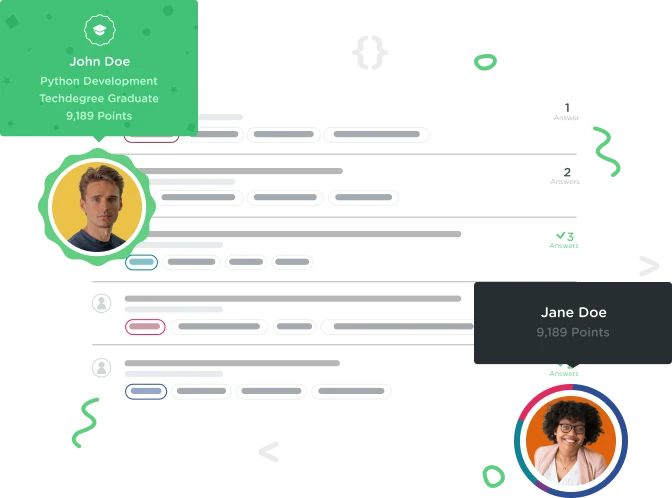

Ken Lawler
163 PointsWhen do you include parentheses on the end of the function name?
In the Capturing Variables section of the Swift Closures course, there is a return statement in which the function name does not have parentheses at the end of it, but elsewhere (ex. when assigning a function to a constant using a let statement), the parentheses. Why the difference? How do we know when to include parentheses?
1 Answer
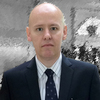
Nathan Tallack
22,160 PointsWhen you are passing a function as a parameter (as is often done when calling a function from another function) you would often use the function name without the brackets because you are not calling the function you are just passing the function as a parameter.
Remember, a function is a first-class type. That means it is just like a variable or a constant or a class object. It can be passed around just as they can. So when you see it being passed around rather than called it will lack the brackets.
Consider the following code.
func makeIncrementer() -> (Int -> Int) {
func addOne(number: Int) -> Int {
return 1 + number
}
return addOne // Here we are returning the function so there is no brackets.
}
var increment = makeIncrementer()
increment(7)
// Excerpt From: Apple Inc. βThe Swift Programming Language.β iBooks.
// https://itunes.apple.com/nz/book/swift-programming-language/id881256329?mt=11
Ken Lawler
163 PointsKen Lawler
163 PointsThanks, Nathan.
Here's the thing: When you write var Increment = makeIncrementer()
You aren't really calling that function, are you?
Nathan Tallack
22,160 PointsNathan Tallack
22,160 PointsYes, you are setting the return value of makeIncrementer to be the value of increment.
But consider the code flow. What is being returned is a function called addOne within the scope of the makeIncrementer function. So that is why you can then get the object you made and pass a parameter into it like the last line does.
Do you understand? In this case increment is not a value type it is a function. So when you declared the variable increment you were really kind of "aliasing" the makeIncrementer addOne function to that variable name.