Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial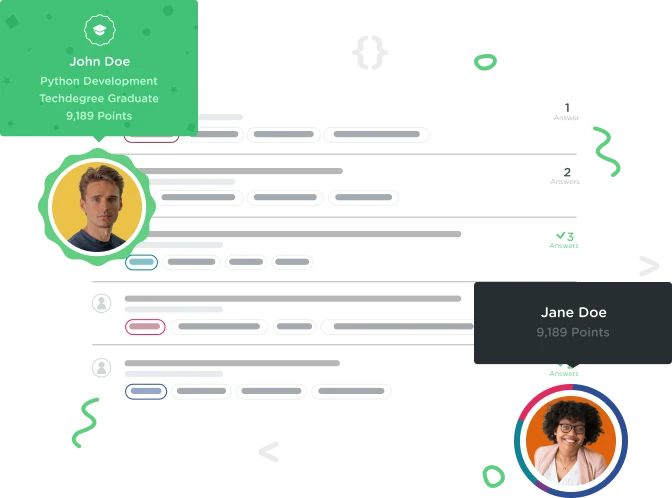

Matthew Egan
1,437 PointsWhen does this not return a value?
I have this set to return a "true" when the numbers repeat, and a "false" in every other situation. Therefore, it should return a value in every scenario. Why then, does it return an error like this? Am I supposed to account for null arrays?
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
int i = 0;
while (i < sequence.Length) {
if ( sequence[i] == sequence[i-1] ) {
return true;
i++;
break;
}
i++;
return false;
}
}
}
}
Oh, and for reference, I used a "While" loop instead of a "foreach" loop because I was getting compiler errors on "for" loops, and while loops are easier to use for array value indications.
4 Answers
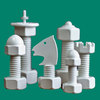
Steven Parker
231,268 PointsThe compiler doesn't know if the loop can never finish, but it sees that there is no return after the loop and complains about that.
But I think you would really want your loop to finish, and only return a false
result at that point.
Also be aware that no statements after a return can ever be executed (like the ones after the return true
), so you can remove them from your code.

Matthew Egan
1,437 PointsThank you! That did the trick.

Matthew Egan
1,437 PointsWait, nevermind, now it says that "index is outside the bounds of the array with this code
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
int i = 0;
while (i <= sequence.Length) {
if ( sequence[i] == sequence[i-1] ) {
i++;
return true;
}
else {
i++;
return false;
}
}
return false;
}
}
}
Any hints on why this is screwing up?
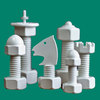
Steven Parker
231,268 PointsThe largest legal index of an array is one less than it's length. So for looping through an array, you want to use index values up to but not equal to the length. But here, you also have the extra complication of comparing each item to the previous one. And anything less than 0 will also not be a valid index, so you may need a different starting value.
And you still have a "return false
" inside the loop.

Matthew Egan
1,437 PointsWell, getting rid of that return false did actually do it. Kind of weird, considering. I was making it so that it would return a value for every value in the array, but I guess I was thinking a little ahead of what the thing needed.