Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial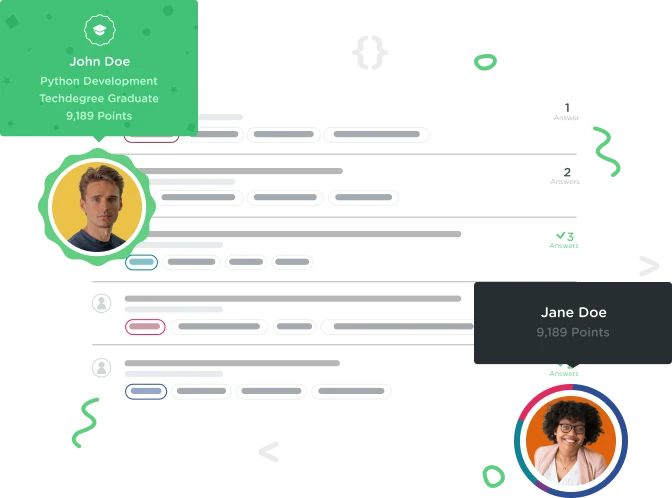

Harrison Court
4,232 PointsWhen ever I try to open the app, it doesn't work...
I'm trying to change the background to red but the app won't even open after! Help me please!
FunFactsActivity.java:
package harrycourt.funfactsapp;
import android.content.DialogInterface;
import android.graphics.Color;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends AppCompatActivity {
private FactBook mFactBook = new FactBook();
// Declare our view vars
private TextView mFactTextView;
private Button mShowFactButton;
private RelativeLayout mRelativeLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign views from the layout file to the corresponding vars
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
mRelativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactBook.getFact();
// Update screen with fact.
mFactTextView.setText(fact);
mRelativeLayout.setBackgroundColor(Color.RED);
}
};
mShowFactButton.setOnClickListener(listener);
}
}
FactBook.java:
package harrycourt.funfactsapp;
import java.util.Random;
/**
* Created by Harry on 1/05/2017.
*/
public class FactBook {
// Fields (Member vars) Propities about the object.
String[] mFacts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built.",
"This app was made possible with the help of Treehouse!" };
// Methods - Actions the object can take
public String getFact() {
String fact = "";
// Randomly select a fact.
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mFacts.length);
fact = mFacts[randomNumber];
return fact;
}
}
activity_fun_facts.xml:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/relativeLayout"
android:background="#51b46d"
tools:context="harrycourt.funfactsapp.FunFactsActivity"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="81dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="40dp"
android:layout_marginStart="16dp"
android:layout_marginTop="40dp"
android:text="Did you know..."
android:textColor="@color/TransparentWhite"
android:textSize="24sp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:layout_constraintLeft_creator="1"
tools:layout_constraintTop_creator="1"/>
<TextView
android:id="@+id/factTextView"
android:layout_width="345dp"
android:layout_height="57dp"
android:layout_marginEnd="8dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="8dp"
android:layout_marginStart="8dp"
android:text="This app was made by the help of Treehouse!"
android:textColor="@android:color/white"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.521"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:layout_constraintBottom_creator="1"
tools:layout_constraintLeft_creator="1"
tools:layout_constraintRight_creator="1"
tools:layout_constraintTop_creator="1"/>
<Button
android:id="@+id/showFactButton"
android:layout_width="0dp"
android:layout_height="55dp"
android:layout_marginBottom="55dp"
android:layout_marginEnd="8dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="8dp"
android:layout_marginStart="8dp"
android:background="@android:color/white"
android:text="Next Fact"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
tools:layout_constraintBottom_creator="1"
tools:layout_constraintLeft_creator="1"
tools:layout_constraintRight_creator="1"/>
</android.support.constraint.ConstraintLayout>
Thanks in advance!

Harrison Court
4,232 PointsI get the simple error in the emulator: "Unfortunately, FunFactsApp has stopped working". But it seems like this is the only error I found:
05-08 22:22:44.508 1064-1064/harrycourt.funfactsapp E/AndroidRuntime: FATAL EXCEPTION: main
java.lang.RuntimeException: Unable to start activity ComponentInfo{harrycourt.funfactsapp/harrycourt.funfactsapp.FunFactsActivity}: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2059)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2084)
at android.app.ActivityThread.access$600(ActivityThread.java:130)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1195)
at android.os.Handler.dispatchMessage(Handler.java:99)
at android.os.Looper.loop(Looper.java:137)
at android.app.ActivityThread.main(ActivityThread.java:4745)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:511)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:786)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:553)
at dalvik.system.NativeStart.main(Native Method)
Caused by: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout
at harrycourt.funfactsapp.FunFactsActivity.onCreate(FunFactsActivity.java:29)
at android.app.Activity.performCreate(Activity.java:5008)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1079)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2023)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2084)
at android.app.ActivityThread.access$600(ActivityThread.java:130)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1195)
at android.os.Handler.dispatchMessage(Handler.java:99)
at android.os.Looper.loop(Looper.java:137)
at android.app.ActivityThread.main(ActivityThread.java:4745)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:511)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:786)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:553)
at dalvik.system.NativeStart.main(Native Method)
1 Answer

Lalit Bagga
3,120 PointsI see the problem.. in your code, you are using relative layout but in XML it is constraint layout. Change your Relative code to this
private ConstraintLayout mConstraintLayout;
mConstraintLayout = (ConstraintLayout) findViewById(R.id.relativeLayout);
mConstraintLayout.setBackgroundColor(Color.RED);

Harrison Court
4,232 PointsThank you so much!
Lalit Bagga
3,120 PointsLalit Bagga
3,120 PointsYou should include error ?? What is the error you are getting ??