Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial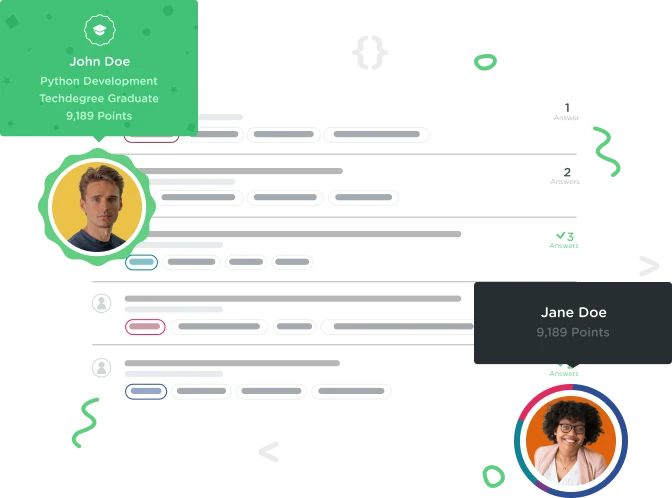

Mark Coup
1,112 PointsWhen guessing non integer, the guess in the formatted clause comes back as an integer?
Whenever I guess a non-integer in this number game, it actually gives me an integer and says it's not a number.
Here's the code:
'''python import random
def game():
# generate a random number between 1 and 10
secret_num = random.randint(1, 10)
guesses = []
# limit number of guesses
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10. "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
# compare the guess to the correct number
if guess == secret_num:
print("You got it! My number was {}.".format(secret_num))
break
# too too message
elif guess < secret_num:
print("My number is higher than {}.".format(guess))
# too high message
else:
print("My number is lower than {}.".format(guess))
guesses.append(guess)
else:
print("You didn't get it, my number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/N ")
if play_again.lower() != "n":
game()
else:
print("Bye!")
game() '''
2 Answers

Sahil Sharma
4,791 PointsThe try part of your code where you take the input from the user has a slight error, you convert/try to convert the input to an integer as soon as the user types in their guess, what you need to account for is that if the user enters something that cannot be turned into an integer then that line of code is redundant as it will not be executed thus if I enter a string, the line of code
guess = int(input("Guess a number between 1 and 10. "))
is not even executed, which means that while executing the .format(guess) in the except part of your code there is no variable existing with the name guess (throwing an error)! So a better structure for using except would be to first accept the input and then try conversion to int. Alternative ->
guess = input("Guess a number between 1 and 10. ")
try:
guess = int(guess)
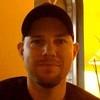
Jeremy Hill
29,567 PointsTry changing the line with your except clause:
#change it from this:
print("{} isn't a number!".format(guess))
#to this:
print("Your entry is invalid! Please use integers only. ")
Mark Coup
1,112 PointsMark Coup
1,112 PointsThanks!