Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial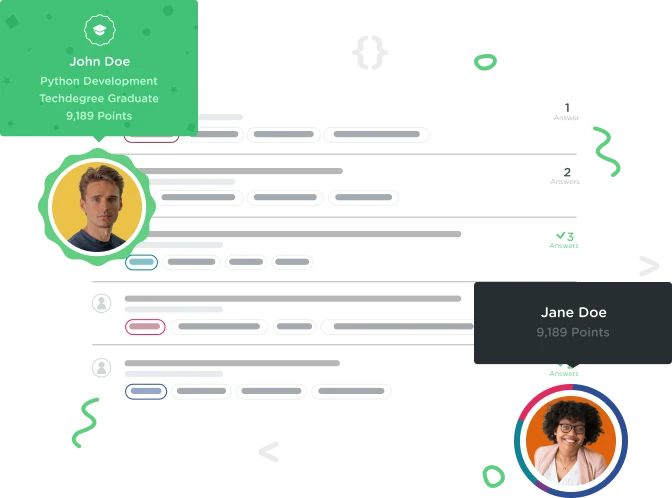

markhsia
2,139 PointsWhen I am compiling my java, it gives me an error: ./PezDispenser.java:2: error: class, interface, or enum expected.
So, it is telling me that I have this error on my second line of code, and is expecting an interface. This is my code with the error symbol:
public static void main(String[] args) { ^ Plus, the error arrow is pointing at the word "void". Can anyone help me?
1 Answer

Benjamin Barslev Nielsen
18,958 PointsYour main method needs to be inside a class with the same name as the file.
public class PezDispenser {
public static void main(String[] args) {
//code to be run
}
}
The error message is pointing at void, since you also can create a public static class, and therefore public static seems right to the compiler, but void is wrong, since the first thing you should do in your java file is define the class, enum or interface.
markhsia
2,139 Pointsmarkhsia
2,139 PointsYes, that worked, but now it says illegal start of expression. This my code: public class PezDispenser{
Benjamin Barslev Nielsen
18,958 PointsBenjamin Barslev Nielsen
18,958 PointsWould you post all your code?
markhsia
2,139 Pointsmarkhsia
2,139 PointsHere: public class PezDispenser { public static void main(String[] args) { public class PezDispenser{ private String mCharacterName; private int mPezCount; public PezDispenser(String CharacterName) { mCharacterName = CharacterName; mPezCount = 0; } public boolean dispense() { boolean wasDispensed = false; if (!isEmpty()) { mPezCount--; wasDispensed = true; } return wasDispensed; } public boolean isEmpty() { return mPezCount == 0; } public final static int MAX_PEZ = 12; public String getCharacterName(){ return mCharacterName; } public void load(){ mPezCount = MAX_PEZ; } } } } Sorry, Don't know how to put in code like you.
Benjamin Barslev Nielsen
18,958 PointsBenjamin Barslev Nielsen
18,958 PointsThe problem is in the beginning of the code:
The main method should only be used if you would execute this class directly. However, none of your code indicates that this is the intention. Therefore I assume that you have a separate class from which you create instances of PezDispenser and uses them. This seperate class then needs to have a main method, but PezDispenser should not have it. The below code should fix the problem:
I have removed the main method and your second class definition, and then you need to remove a couple of } at the end of the file.
markhsia
2,139 Pointsmarkhsia
2,139 PointsI still don't understand why you took out the public static void main(String[] args) { ps: how do you put in code like that?
Benjamin Barslev Nielsen
18,958 PointsBenjamin Barslev Nielsen
18,958 PointsCode can be but in by using
```java
Your code
```
If you write in another language, you can replace java with that language.
The main method is what is run when you run the statement (assuming you have compiled it):
java PezDispenser
However when you have a Java project you usually have multiple files that work together, but the user should only start one program (the main program), and then the other java files are used by the main program. Therefore only the main program should have a main method. Consider this Driver for the PezDispenser:
We will now run our program (again assumed it is compiled), which uses our PezDispenser:
java Driver
Since we use this Driver, we do not want to run PezDispenser for itself, and therefore we should not have a main method inside it.
I hope it makes sense
markhsia
2,139 Pointsmarkhsia
2,139 PointsI'm so sorry, but I still don't understand. Thanks a lot anyways.