Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial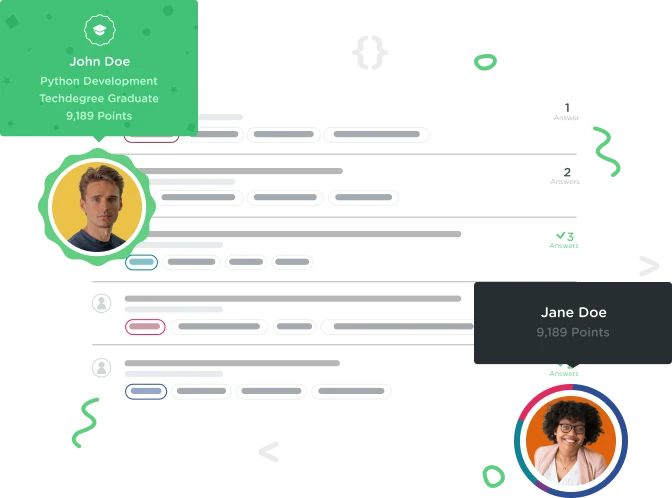

Chantal St Louis
5,524 PointsWhen I answer question 4 correctly, my score doesn't increase? I also get 'undefined' when my score is 0? Thanks
/*
- Store correct answers
- When quiz begins, no answers are correct */
let score = 0;
// 2. Store the rank of a player let rank;
// 3. Select the <main> HTML element const main = document.querySelector('main');
/*
- Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers */
const question1 = prompt('Is Chan allergic to cats?'); if( question1.toUpperCase() === 'YES') { score += 1; } console.log(question1); console.log(score);
const question2 = prompt('Is Chan allergic to all dogs'); if( question2.toUpperCase() === 'NO'){ score += 1 } console.log(question2); console.log(score);
const question3 = prompt('Does Chan prefer crisps over sweets'); if( question3.toUpperCase() === 'YES' ) { score += 1; } console.log(question3); console.log(score);
const question4 = prompt('Is Chan\'s mum\'s name Lynn?'); if(question4.toUpperCase() === 'YES') { score += 1; } console.log(question4); console.log(score);
const question5 = prompt('How many years has Steve been with Gail?'); if(question5.toUpperCase() === 'two' || question5 === 2 ) { score += 1; } console.log(question5); console.log(score);
/*
- Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown */
if( score === 5 ) { rank = 'Gold' } else if( score === 3 || score === 4 ) { rank = 'Silver' } else if( score === 1 || score === 2) { rank = 'Bronze' } if ( score === 0 ){ 'Sorry no crown...' }
// 6. Output results to the <main> element
main.innerHTML = <p>Your score was ${score} so your rank is ${rank}! </p>
1 Answer
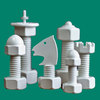
Steven Parker
231,269 PointsThis test can never be true:
if (question5.toUpperCase() === 'two' || question5 === 2 )
Anything converted to upper case can never equal "two", and since question5 is a string, the strict comparison operator will not consider it equal to the number 2. You may want to write it like this instead:
if (question5.toUpperCase() === 'TWO' || question5 === "2")
Then, "rank" was never declared so unless it gets implicitly declared global by assignment (which it does not when the score is 0) then it will be undefined.
And when posting code to the forum, use Markdown formatting to preserve the appearance, and optionally add syntax coloring (as I did here).
Eduard Edwin
14,569 PointsEduard Edwin
14,569 PointsAdding on to what Steven said, there is no else statement after the second else-if statement. The syntax should be:
The variable "rank" is not declared. This should be done in step 2.