Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial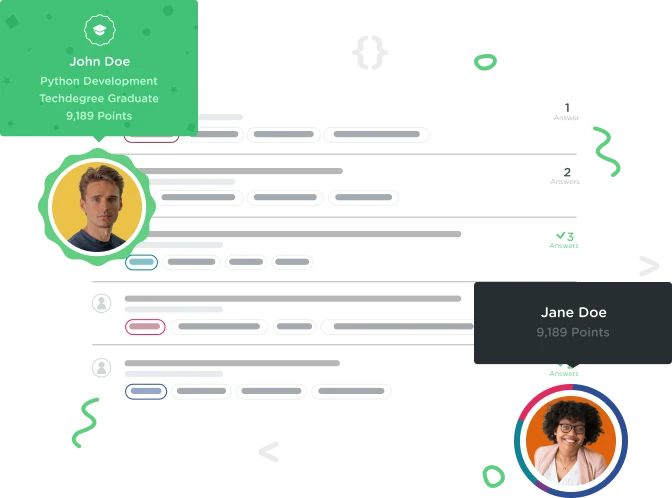

Terrell Stewart
Courses Plus Student 4,228 PointsWhen I attempt to create a taco I get a peewee.OperationalError: table taco has no column named timestamp
When I attempt to create a taco I get a peewee.OperationalError: table taco has no column named timestamp
so I tried deleting the timestamp to see if this would affect anything and I received a peewee.OperationalError: table taco has no column named user_id
Why is the program behaving this way this time?
import datetime
from peewee import *
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
DATABASE = SqliteDatabase('memory.db')
#Make User
class User(UserMixin,Model):
email = CharField(unique=True)
password = CharField(max_length=100) #Give the User an email and a passsword
class Meta:
database = DATABASE
@classmethod #This makes cls == User class find out the WHY
def create_user(cls,email,password, admin=False):
try:
with DATABASE.transaction():
cls.create(
email = email,
password=generate_password_hash(password),
is_admin=admin #Provide a create User method,that hashes their passwords
)
except IntegrityError:
raise ValueError('User already exists')
class Taco(Model):
timestamp = DateTimeField(default=datetime.datetime.now)
user = ForeignKeyField(
rel_model=User,
related_name='tacos'
)
protein = CharField(max_length=25)
shell = CharField(max_length=25)
cheese = BooleanField()
extras = TextField()
class Meta:
database = DATABASE
order_by = ('-timestamp',)
#Provide a create User method,that hashes their passwords
def initialize():
DATABASE.connect()
DATABASE.create_tables([User,Taco],safe=True)
DATABASE.close()
2 Answers

Terrell Stewart
Courses Plus Student 4,228 PointsNever mind I figured out that it was due to not having a create method within my Taco class that I was trying to access through my view
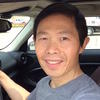
David Lin
35,864 PointsAnother way to fix similar peewee issues:
Delete the database .db file.
If the datatbase file was created and afterwards you modified or added any model fields, then the new fields won't be recognized in the database. Deleting the .db file will recreate a new one on start-up and fix the issue.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsMoved comment to answer.
David Lin
35,864 PointsDavid Lin
35,864 PointsShouldn't need to explicitly write a create method for models, unless you want a custom initializer, so doesn't sound like that was the true cause of your error.