Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial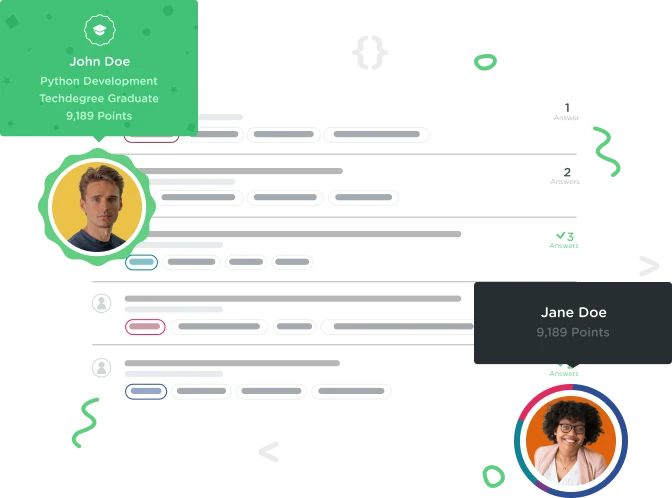

Anam Hira
6,444 PointsWhen I Execute in Console Nothing Happens.
When I press execute the code nothing happens and goes to the next line.
Cookbook.php
<?php
include("classes/recipe.php");
include("classes/render.php");
include("inc/allrecipes.php");
include("classes/recipecollection.php");
$cookbook = new RecipeCollection("Treehouse Recipes");
$cookbook->addRecipe($lemon_chicken);
$cookbook->addRecipe($granola_muffins);
$cookbook->addRecipe( $belgian_waffles);
$cookbook->addRecipe( $pepper_casserole);
$cookbook->addRecipe($lasagna);
$cookbook->addRecipe($dried_mushroom_ragout);
$cookbook->addRecipe($rabbit_catalan);
$cookbook->addRecipe($grilled_salmon_with_fennel);
$cookbook->addRecipe($pistachio_duck);
$cookbook->addRecipe($chili_pork);
$cookbook->addRecipe($crab_cakes);
$cookbook->addRecipe($beef_medallions);
$cookbook->addRecipe($silver_dollar_cakes);
$cookbook->addRecipe($french_toast);
$cookbook->addRecipe($corn_beef_hash);
$cookbook->addRecipe($granola);
$cookbook->addRecipe($spicy_omelette);
$cookbook->addRecipe($scones);
$breakfast = new RecipeCollection("Breakfeasts");
foreach ($cookbook->filterByTag("breakfeast") as $recipe) {
$breakfast->addRecipe($recipe);
}
echo Render::listRecipes($breakfast->getRecipeTitles());
//echo Render::displayRecipe($lemon_chicken);
Render.php
<?php
class Render
{
public function __toString()
{
$output = "The following methods are available for " . __CLASS__ ." objects: \n";
$output .= implode("\n", get_class_methods(__CLASS__));
return $output;
}
public static function listIngredients($ingredients)
{
$output = "";
foreach ($ingredients as $ing) {
$output .= $ing["amount"] . " " . $ing["measure"] . " " . $ing["item"];
$output .= "\n";
}
return $output;
}
public static function displayRecipe($recipe)
{
$output = "";
$output .= $recipe->getTitle() . " by " . $recipe->getSource();
$output .= "\n";
$output .= implode(", ",$recipe->getTags());
$output .= "\n\n";
$output .= self::listIngredients($recipe->getIngredients());
$output .= "\n";
$output .= implode("\n", $recipe->getInstructions());
$output .= "\n";
$output .= $recipe->getYield();
return $output;
}
public static function listRecipes($titles)
{
return implode("\n",$titles);
}
}
Recipe Collection.php
<?php
class RecipeCollection
{
private $title;
private $recipes = array();
public function __construct($title = null)
{
$this->setTitle($title);
}
public function setTitle($title)
{
if(empty($title)) {
$this->title = null;
} else{
$this->title = ucwords($title);
}
}
public function getTitle()
{
return $this->title;
}
public function addRecipe($recipe)
{
$this->recipes[] = $recipe;
}
public function getRecipes()
{
return $this->recipes;
}
public function getRecipeTitles()
{
$titles = array();
foreach ($this->recipes as $recipe) {
$titles[] = $recipe->getTitle();
}
return $titles;
}
public function filterByTag($tag)
{
$taggedRecipes = array();
foreach($this->recipes as $recipe) {
if (in_array(strtolower($tag), $recipe->getTags())){
$taggedRecipes[] = $recipe;
}
}
return $taggedRecipes;
}
}
Recipe.php
<?php
class Recipe
{
private $title;
private $ingredients = array();
private $instructions = array();
private $yield;
private $tag = array();
private $source;
private $measurements = array(
"tsp",
"tbsp",
"cup",
"oz",
"lb",
"fl oz",
"pint",
"quart",
"gallon"
);
public function __construct($title = null)
{
$this->setTitle($title);
}
public function __toString()
{
$output = "You are calling a " . __CLASS__ . " object with the title \"";
$output.= $this->getTitle() . "\"";
$output.= "\nIT is stored in " . basename(__FILE__) . " at" . __DIR__ . ".";
$output.= "\nThis display is form line" . __LINE__ . "in method" . __METHOD__;
$output.="\nThe following methods are available for objects on this class:\n" ;
$output.= implode("\n",get_class_methods(__CLASS__));
return $output;
}
public function setTitle($title)
{
if(empty($title)) {
$this->title = null;
} else{
$this->title = ucwords($title);
}
}
public function getTitle()
{
return $this->title;
}
public function addIngredient($item, $amount=null, $measure = null)
{ if ($amount != null && !is_float($amount) && !is_int($amount)) {
exit("The amount must be a float: " . gettype($amount) . "Amount Given");
}
if ($measure !=null && !in_array(strtolower($measure), $this->measurements)) {
exit("Please enter a valid measurement:" . implode(", ", $this->measurements));
}
$this->ingredients[] = array(
"item" => ucwords($item),
"amount" => $amount,
"measure" => strtolower($measure)
);
}
public function getIngredients()
{
return $this->ingredients;
}
public function addInstruction($string)
{
$this->instructions[] = $string;
}
public function getInstructions()
{
return $this->instructions;
}
public function addTag($tag)
{
$this->tags[] = strtolower($tag);
}
public function getTags()
{
return $this->tags;
}
public function setYield($yield)
{
$this->yield = ucwords($yield);
}
public function getYield()
{
return $this->yield;
}
public function setSource($source)
{
$this->source = ucwords($source);
}
public function getSource()
{
return $this->source;
}
public function displayRecipe()
{
return $this->title . " by " . $this->source;
}
}
1 Answer

Greg Kaleka
39,021 PointsHi Anam,
You have the wrong filename being included: you want to include classes/recipes.php, not classes/recipe.php.
Cheers
-Greg
Anam Hira
6,444 PointsAnam Hira
6,444 PointsThank You
-Anam