Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial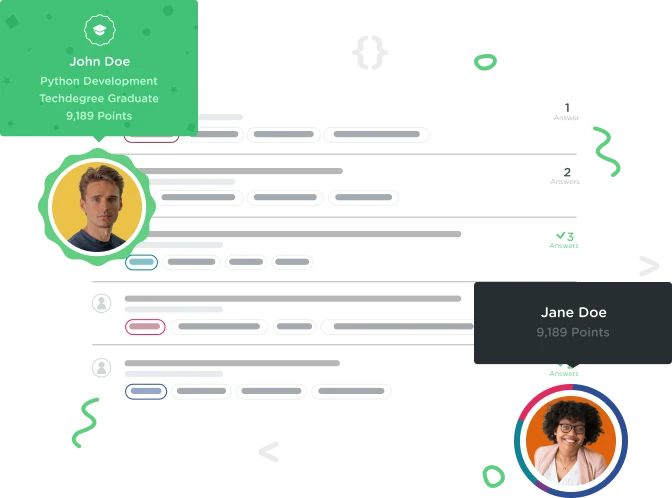

Iver Band
8,389 PointsWhen I hide an HTML element at the start of my script, I can still see it for an instant when it loads. Any fixes?
For example,
I can still see the spoiler text briefly even after developing a correct app.js script that hides the text and then shows the text and hides the button when the button is clicked.
The same was true for the flash message in the previous project. I could see it briefly whenever I reloaded the page.
I am using a fairly powerful tower PC, Windows 10, and the latest version of chrome.
7 Answers

Michael Schut
739 PointsThe reason you can see it for a small amount of time is because the page is still loading. The way a webpage loads is top to bottom. If you have the "<script src="app.js"></script>" at the bottom of the <body> then that will be the last item on the page to load. It is waiting for everything else to load in then it will process the javascript.
Another possible answer is if you have a "$(window).load();" surrounding your javascript. That means that every single element on the page will load before running the javascript inside the load callback.
Hope this answers your question!
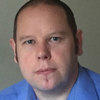
Alan McClenaghan
Full Stack JavaScript Techdegree Graduate 56,501 PointsIn the CSS file, change .spoiler span
to { display: none; }
.
This will prevent the spoiler being revealed but will mean the span element has no padding applied.
Then in the JavaScript, rather than use the .show()
method, you should instead use .css('display', 'block')
to change the css property value back to block.

David Toops
19,313 PointsI realize it's been over a year since this question was posted -- but I didn't see the suggestion of loading the span w/o text and inserting it when the button is pressed.
From index.html <p class="spoiler"> <span></span> <button>Reveal Spoiler</button> </p>
from app.js const spoilerText = "Darth Vader is Luke Skywalker's Father! Noooooooooooo!"
$('.spoiler button').click(function(){ //Show the spoiler text $('.spoiler span').text(spoilerText); $('.spoiler span').show(); //Hide the "Reveal Spoiler" button $('.spoiler button').hide(); });

Iver Band
8,389 PointsThanks, Michael. I guess the fix would be to code the HTML to hide the content, and use JavaScript/JQuery to unhide it.

Kate C
13,589 PointsI have the same question as what Dominykas Kuizinas has asked. Why move the <script> to the top of the <body> element or inside (head> doesn't run at all?? If they can be load first, isn't the problem would be solved?
I know it is better to put <script>at the bottom before <body>, but a small project like this, i guess it is ok???

Ossian Nörthen
5,518 PointsHere's my solution to fix the spoiler showing on load. Maybe it's helpful to someone.
HTML: <!DOCTYPE html> <html> <head> <title>Star Wars Spoilers</title> <link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8"> </head> <body> <img src="img/deathstar.png" /> <div class="spoiler"> <span>Darth Vader is Luke Skywalker's Father! Noooooooooooo!</span> </div> <div class="button"> </div>
<script src="js/jquery-3.2.1.min.js" type="text/javascript" charset="utf-8"></script> <script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body> </html>
APP.JS: const spoiler = $('.spoiler'); const buttonDiv = $('.button'); const $button = $('<button>Reveal the spoiler</button>');
buttonDiv.append($button)
$button.click(function(){ spoiler.slideDown(1000); })
CSS: body { background: #2f558e url(../img/bg.png) repeat 0 0; background-size: 400px auto; font-family: sans-serif; } img { display: block; width: 150px; margin: 100px auto; } button { background: #dae1e4; border: none; border-radius: 5px; color: #1d3c6a; font-size: 24px; width: 480px; padding: 40px 0; margin: 0px -20px; outline: none; cursor: pointer; } .button { background: #1d3c6a; width: 440px; margin: 0 auto 20px; border-radius: 5px; text-align: center; font-size: 24px } .spoiler { background: #1d3c6a; width: 440px; margin: 0 auto 20px; border-radius: 5px; text-align: center; font-size: 24px; display:none; } .spoiler span { color: #dae1e4; padding: 40px 20px; display:inline-block; }

Tomas Vesely
Full Stack JavaScript Techdegree Graduate 25,309 PointsI created a "hidden" class attribute in CSS. Which I appended to a span. css: .hidden { display: none;}
html: <span class="hidden">Darth Vader is Luke Skywalker's Father! Noooooooooooo!</span>
That way the span will not show at the very begining.
Then then I found removeClass() method. When a button is clicked, "hidden" class is removed and a span pops up.
Dominykas Kuizinas
10,761 PointsDominykas Kuizinas
10,761 PointsSo is there simple way to fix it ? I'v tried to place <script...> in the top of the <body> element, but then app.js doesn't run at all. Thanks in advance