Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial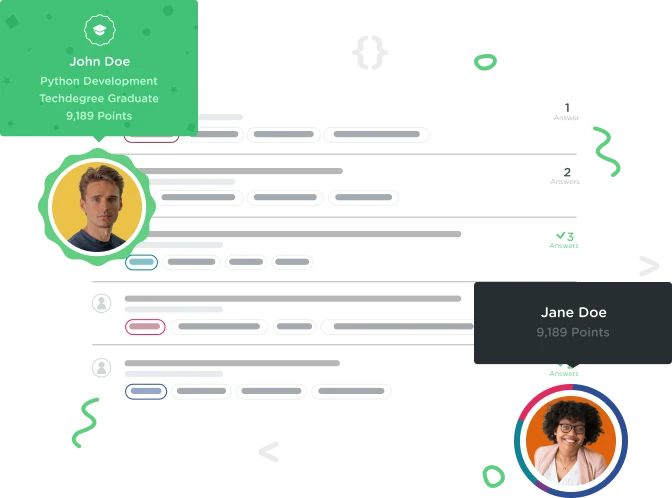

William Ma
Courses Plus Student 1,659 PointsWhen I launch my code, i end up with an "html" body rather than what should be displayed.
//Problem: we need a simple way to look at a user's badge count and javascript points
//Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
var http = require("http");
var username = "williamma2";
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in JavaScript";
console.log(message);
}
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username.json, function(response){
var body = "";
//Read the Data
response.on('data', function (chunk){
body += chunk;
});
response.on('end', function(){
console.log(body);
console.log(typeof body);
});
//Parse the data
//Print the data
});
request.on("error", function(error){
console.error(error.message);
});
I've been trying to type in Sublime and use the mac terminal as the console.
3 Answers
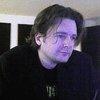
Rick Hoekman
9,494 PointsI think you need to make sure that the .json is actually added to the url:
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response)

William Ma
Courses Plus Student 1,659 PointsThanks Rick, It worked after that fix. I wonder why it returned something different...
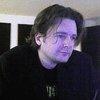
Rick Hoekman
9,494 PointsYou're welcome :)
Well you still made a http GET request so you got a response back from the webserver. Even if the page does not exist you will normally get a response (404 error) back from the webserver.
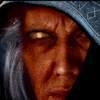
Raistlin Majere
Courses Plus Student 3,425 PointsI know this is late but, for any others that might have this question, the reason you got HTML back is that the .json part was not correctly concatenated into the GET request. In the example above the student attempted to pass in a variable which was undefined (username.json) so what was sent in the HTTP request was http://teamtreehouse.com/undefined. So the response was not a JSON file but rather an HTML file, which was subsequently returned and assigned to the variable body, which was then logged to the console.
If you go to http://teamtreehouse.com/ you will see the HTML which was passed back.