Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial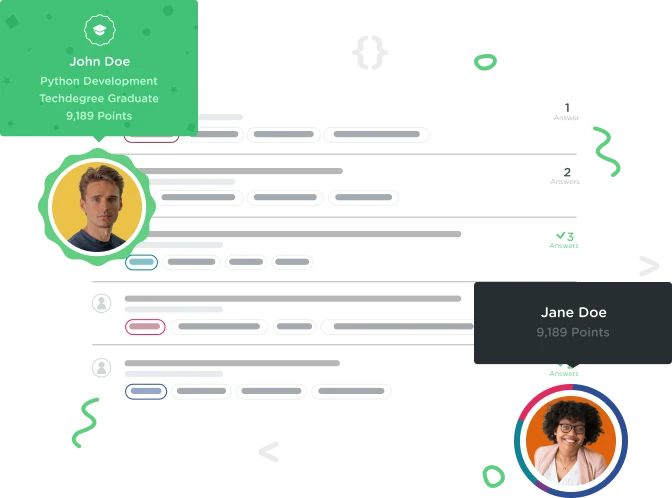

Wannabe Engineer
9,186 PointsWhen I run the app I get my dialog error message("Oops sorry - there was an error, please try again!")
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "*** AN API KEY ***";
double latitude = 37.8267;
double longtitude = -122.4233;
String forecastUrl = "https://api.darksky.net/forecast/" + apiKey +
"/," + latitude + "," + longtitude;
if (isNetworkEnable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
Log.v(TAG, response.body().string());
if (response.isSuccessful()) {
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught:", e);
}
}
});
}
else{
Toast.makeText(this, R.string.network_unavailable_message,Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running!");
}
private boolean isNetworkEnable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
alertDialogFragment dialog = new alertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
This error should pop up if -> try { Log.v(TAG, response.body().string()); doesn't go well and else statement runs instead of >if< statement, so whats wrong with the response.body().string()
2 Answers

Wannabe Engineer
9,186 PointsI fixed it :d In the String forecastUrl > +"/," is incorrect, Comma is not needed.
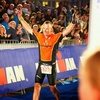
Steve Hunter
57,712 PointsGlad you got it fixed. Apologies for the lack of response. The usual notification emails didn't arrive for some reason.
Steve.

Wannabe Engineer
9,186 PointsThanks for editing Steve, in this course , we built a Dialog message , which was going to pop up when u ran an app and something went wrong, we stored that dialog message in alertUserAboutError() method, and that method creates a new object of AlertDialogFragment . The problem is that , I dont see any reason why this message should pop-up , I can't find any errors .
public class alertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setTitle(R.string.titleMessage)
.setMessage(R.string.errorMessage)
.setPositiveButton(R.string.buttonText,null);
AlertDialog dialog = builder.create();
return dialog;
}
}
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI edited your code block and hid your API key.
I'm not clear on the question, though. What line is throwing the error, or where is your dialog error message coming from? What's the precise problem here?
Steve.