Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial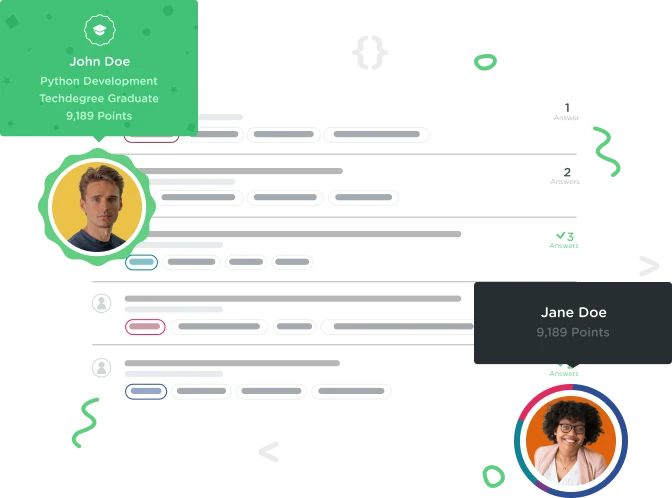

MUZ140753 Nyaradzai Matiza
6,064 PointsWhen I run the console, I get this error message: Uncaught TypeError: Cannot read property 'parentNode' of undefined.
here is my full code
//Problem: User interraction doesnt provide required results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button on the page
var incompleteTaskHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//New task list item
var createNewTaskElement = function(taskString) {
//Create list Item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input");
//label
var label = document.createElement("label");
//input(text)
var editInput = document.createElement("input");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//each element needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
editButton.className = "delete";
label.innerText = taskString;
//each element needs to be appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//Create a new list item with the text from #new task
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to the incompleteTaskHolder
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...");
//When the Edit button is pressed
//if the class of the parent is .editMode
//Switch from .editMode
//label text become the input's value
//else
//Switch to .editMode
//input value becomes the label's text
//toggle .editMode on the parent
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//Remove the parent list item from the ul
ul.removeChild(listItem);
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Task Complete...");
//Append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//Append the task list item to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler){
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask();
//bind deleteTask to delete button
deleteButton.onclick = deleteTask();
//bind taskCompleted to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the add task function
addButton.onclick = addTask;
var ajaxRequest = function(){
console.log("AJAX request");
}
addButton.onclick = ajaxRequest;
//Cycle over the incompleteTaskHolder ul list items
for (var i = 0; i < incompleteTaskHolder.children.length; i++) {
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i], taskCompleted);
}
//Cycle over the completeTaskHolder ul list items
for (var i = 0; i < completedTasksHolder.children.length; i++) {
//bind events to list item's children (taskInComplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
1 Answer
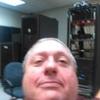
Calvin Miracle
22,028 PointsHi there,
Yes sure enough, your variable 'parentNode' is not defined. It does not show up on the LEFT side of an assignment anywhere in your example code.
Somewhere, somehow, 'parentNode' needs to be declared into existence before it can be used for later work
I hope this helps, take care!
-- Cal
Jonathan Leon
18,813 PointsJonathan Leon
18,813 PointsCould you please copy it again from the workspaces and paste it between
three ticks (`)javascript
code here
three ticks (`)