Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial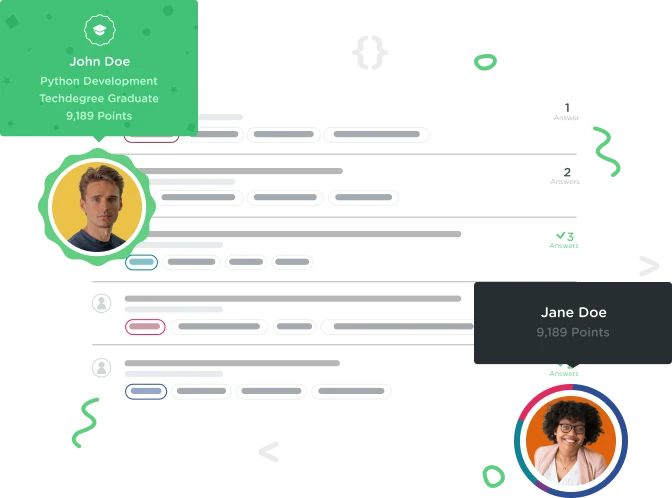
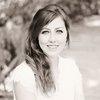
Meg Seegmiller
15,150 PointsWhen I submit task 1, it passes; but after adding my code for task 2, I receive an error that Task 1 no longer passes
When I submit task 1, it passes; but after adding my code for task 2, I receive an error that Task 1 no longer passes even though no changes have been made to the code for Task 1. Help!
1st Challenge:
Move the 1 to position 0. You can do this in one step with .pop() and .insert().
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0,the_list.pop(3))
2nd Challenge:
Use .remove() and/or del to remove the string, boolean, and list members of the_list.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0,the_list.pop(3))
i=0
for item in the_list:
del the_list[i]
the_list.remove(item)
i+=1
del the_list[i]
del the_list
2 Answers
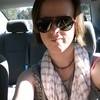
karis hutcheson
7,036 PointsIt's because, in the second section of your code, you basically delete everything in the list. So the checker looks for "1" to be in position "0" and doesn't find it. It then stops looking and says that task one no longer passes. Technically, both challenges are failing.
What he's basically asking for is to remove indexes 1, 4 and 5 (after the move) which correspond to the "a", False and [1,2,3] list. You can either accomplish this by using del to delete their indexes (not a great method, but it will pass you):
del the_list[5]
del the_list[4]
del the_list[1]
or using .remove() to get them (better way):
the_list.remove("a")
the_list.remove(False)
the_list.remove([1,2,3])
Remember, when using the del method: I did them from last index to first because Python parses code one line at a time. Meaning that if you del [1] first, all of the other items' indexes change where 2 becomes 1, 3 becomes 2 and so forth.
What you did in your for loop was to go through every item and delete them. You ended with an empty list, then called del the_list which killed the variable in memory too.

Ken Alger
Treehouse TeacherMegan;
Typically when the challenge engine errors out like that it means there is a syntax error occurring preventing it from working at all.
Having said that, I posted a response on this same challenge earlier today. Take a look and see if it helps.
Happy coding,
Ken
Meg Seegmiller
15,150 PointsMeg Seegmiller
15,150 PointsThis is what I get for trying to do the code challenge late at night! I was reading the question as removing all the items from the list rather than just the three the challenge lists.
And thank you for explaining how my errors in challenge two affected challenge one.
karis hutcheson
7,036 Pointskaris hutcheson
7,036 PointsNo prob! :)