Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial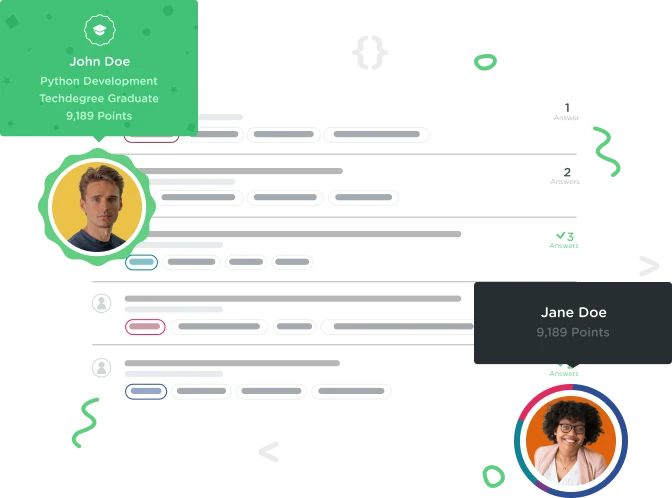

Phillip Chan
3,914 PointsWhen I try to create a new user, it gives the error "User.create is not a function”.
I get this error when I try to create a new user at this step. I’m not sure if Mongoose is different than yours but this is my code. It seems the same as the instructors.
var express = require('express'); var router = express.Router(); var User = require('../models/user');
// GET /
router.get('/', function(req, res, next) {
return res.render('index', { title: 'Home' });
});
// GET /about
router.get('/about', function(req, res, next) {
return res.render('about', { title: 'About' });
});
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});
router.get('/register', function(req, res, next) {
return res.render('register', {title: 'Sign Up'});
});
router.post('/register', function(req, res, next) {
// make sure
if (req.body.name &&
req.body.email &&
req.body.favoriteBook &&
req.body.password &&
req.body.confirmPassword) {
if (req.body.password !== req.body.confirmPassword) {
var err = new Error("passwords do not match");
err.status = 400;
return next(err);
}
else {
// it works!
// create the object we're going to send the mongoose
var userData = {
email: req.body.email,
name: req.body.name,
favoriteBook: req.body.favoriteBook,
password: req.body.password
};
// insert into mongo with Schema's create method from mongoose
User.create(userData, function(error, user) {
if (error) {
return next(error);
} else {
// follow up action
return res.redirect('/profile');
}
});
}
} else {
var err = new Error('All fields required');
err.status = 400;
return next(err);
}
});
module.exports = router;
4 Answers

Phillip Chan
3,914 PointsNvm, there was a syntax error
should’ve been
module.exports = User;
Thanks for your help everyone!

Marie Nipper
22,638 PointsHa. Nevermind. You got it. :)

Donnie Reese
17,211 PointsHuzzah!
In your module, when you do the export.
You have set the variable module.export to User.
var User = mongoose.model("User", UserSchema);
module.export = User;
where you need to add your User to module.export.
var User = mongoose.model("User", UserSchema);
module.export.User = User;
This tells it that you want to export the User variable. That could be named whatever you wish.

Donnie Reese
17,211 PointsThe problem may be in the User scheme from the model. It says that that function doesn't exist, not the actual exported User.
It may be that it wasn't exported from the model correctly, but I am really just guessing in the dark.

Phillip Chan
3,914 PointsSorry, here is the user.js!
var mongoose = require('mongoose');
var UserSchema = new mongoose.Schema({
email: {
type: String,
unique: true, // no two users can create two same emails
required: true,
trim: true // removes whitespace accidentally
},
name: {
type: String,
unique: false,
required: true,
trim: true
},
favoriteBook: {
type: String,
unique: false,
required: true,
trim: true
},
password: {
type: String,
required: true,
trim: false
}
});
var User = mongoose.model("User", UserSchema);
module.export = User;

Marie Nipper
22,638 PointsYou module.export line should read module.exports You have to make export plural.
Marie Nipper
22,638 PointsMarie Nipper
22,638 PointsFrom your models folder, can you post your user.js file?