Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial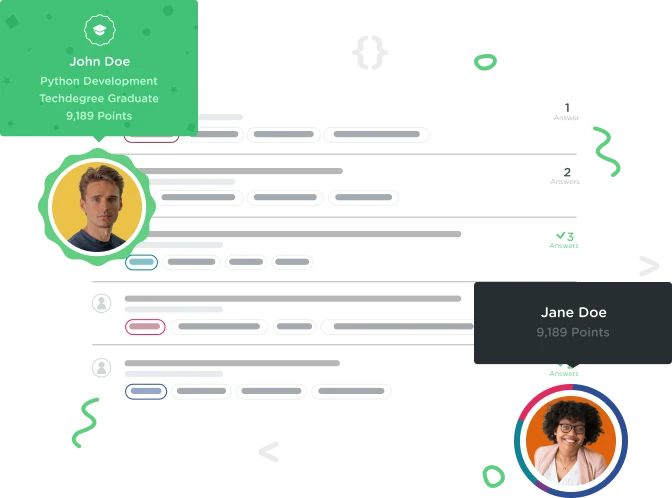

Mads Bennicke
2,630 PointsWhen I try to run VendingMachine the debunk area says 2016-09-08 20:06:22.241 VendingMachine[3377:267843] Failed to
VendingMachine.swift
// // VendingMachine.swift // VendingMachine // // Created by Mads on 05/09/2016. // Copyright © 2016 Treehouse. All rights reserved. //
import Foundation
// Protocols
protocol VendingMachineType { // VendingMachine'en var selection: [VendingSelection] { get } // den skal have et vare udvalg var inventory: [VendingSelection: ItemType] { get set } // der skal være et ental vare var amountDeposited: Double { get set } // der skal være nogle penge imaskinen at købe for
init(inventory: [VendingSelection: ItemType]) // den skal initieres så der er nogle vare i den
func vend(selection: VendingSelection, quantity: Double) throws // den skal have en vend func der skal bruge en vare udvalg og en antal vare
func deposit(amount: Double) // den skal have en deposit func som skal bruge en amount
}
protocol ItemType { // alle vare typer var price: Double { get } // skal have en pris var quantity: Double { get set } // skal have et antal }
// Error Types
enum InventoryError: ErrorType { case InvalidResouce case ConvertionError case InvaledKey }
// Helper Classes
class PlistConverter { class func dictionaryFromFile(resource: String, ofType type: String) throws -> [String : AnyObject] {
guard let path = NSBundle.mainBundle().pathForResource(resource, ofType: type) else {
throw InventoryError.InvalidResouce
}
guard let dictionary = NSDictionary(contentsOfFile: path),
let castDictionary = dictionary as? [String: AnyObject] else {
throw InventoryError.ConvertionError
}
return castDictionary
}
}
class InventoryUnarchier { class func vendingInventoryFromDictionary(dictionary: [String : AnyObject]) throws -> [VendingSelection : ItemType] {
var inventory: [VendingSelection : ItemType] = [:]
for (key, value) in dictionary {
if let itemDict = value as? [String : Double],
let price = itemDict["price"], let quantety = itemDict["quantety"] {
let item = VendingItem(price: price, quantity: quantety)
guard let key = VendingSelection(rawValue: key) else {
throw InventoryError.InvaledKey
}
inventory.updateValue(item, forKey: key)
}
}
return inventory
}
}
// Concrete Types
enum VendingSelection: String { // her er vare udvalget case Soda case DietSoda case Chips case Cookie case Sandwich case Wrap case CandyBar case PopTart case Water case FruitJuice case SportsDrink case Gum }
struct VendingItem: ItemType { // vare der ken sælgges skal følge ItemType protocol'en og skalderfor have let price: Double // en pris var quantity: Double // et antal }
class VendingMachine: VendingMachineType { // VendingMachine skal opfylde alle kravendei VendingMachineType let selection: [VendingSelection] = [ // derfor skal den have et udvalg af vare .Soda, .DietSoda, .Chips, .Cookie, .Sandwich, .Wrap, .CandyBar, .PopTart, .Water, .FruitJuice, .SportsDrink, .Gum]
var inventory: [VendingSelection : ItemType] // derfor skal den have en mængde af vare og det skal være varende fra VendingSelection med tilhørende antal og priser somdet er spesifiseret i Itemtype
var amountDeposited: Double = 10 // derfor skal den have noglepenge at buge af
required init(inventory: [VendingSelection : ItemType]) { // det er også spesifisret at den skal initialiseres med et vare antal så der er noget at sælge af
self.inventory = inventory
}
func vend(selection: VendingSelection, quantity: Double) throws {
// add code
}
func deposit(amount: Double) {
// add code
}
}
VendingItemCell.swift
// // VendingItemCell.swift // VendingMachine // // Created by Pasan Premaratne on 1/19/16. // Copyright © 2016 Treehouse. All rights reserved. //
import UIKit
class VendingItemCell: UICollectionViewCell {
@IBOutlet weak var iconView: UIImageView!
}