Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial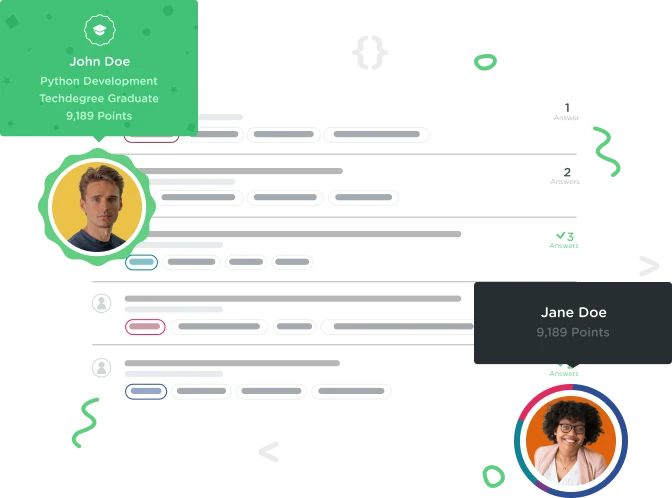

DC Cruce
1,997 PointsWhen I write a function between the script tags it tells me that Task 1 s no longer passing.
The opening and closing script tags have not been modified at all. The function is written on it's own set of indented lines which fall inside the opening and closing script tags.
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script>
function alert() {
alert('Warning!);
}
</script>
</body>
</html>
2 Answers
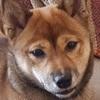
Katie Wood
19,141 PointsHi there,
Nothing wrong with Task 1 - the reason you're getting that message is that there is an error inside the <script> tags, which is causing it to stop before getting to the closing </script> tag. Because of that, it's failing Task 1 but the actual problem is in the JavaScript.
There are a couple things inside the script. First, the function you create cannot be called "alert" here - alert is already a built-in JavaScript function, so it's going to throw an error when you try to use it. Second, the reason it's throwing a syntax error right now is because the string inside the alert('Warning!); is missing a closing quote. Finally, you need to call your function in the script so that it will actually run.
Broken down:
<script>
function alert() { //call it something else, e.g. sendAlert
alert('Warning!); //add closing quote
}
//call your function, e.g. sendAlert();
</script>
That should do it. Good luck!

DC Cruce
1,997 PointsI appreciate your response. I resolved this a little bit ago by starting the video over, redoing the steps in the video and then tackling the problem with a more or less "clean slate". I appreciate your timely response. I will try to remember that alert is a built-in JavaScript function.
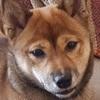
Katie Wood
19,141 PointsGlad you got it! Luckily, you don't really need to memorize the built-in functions - it's more like something to keep in mind if you run into errors or things aren't behaving the way you expect. It won't even necessarily throw an error all the time - in this case, it would because you also call the actual alert() function inside of your alert() function - it causes a "Too much recursion" error because it thinks the function is calling itself, since they have the same name. Just generally something to avoid.
If you're interested in testing it out, you can see the recursion error by going into the javascript console in the browser (ctrl+shift+j in Chrome, ctrl+shift+k in Firefox) and typing:
function alert() { alert("Warning!") }
followed by
alert()
However, if you make it something like
function alert() { return "You have been alerted!" }
and then call alert(), it will return the value. However, the normal alert() won't work until you reset the console.