Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial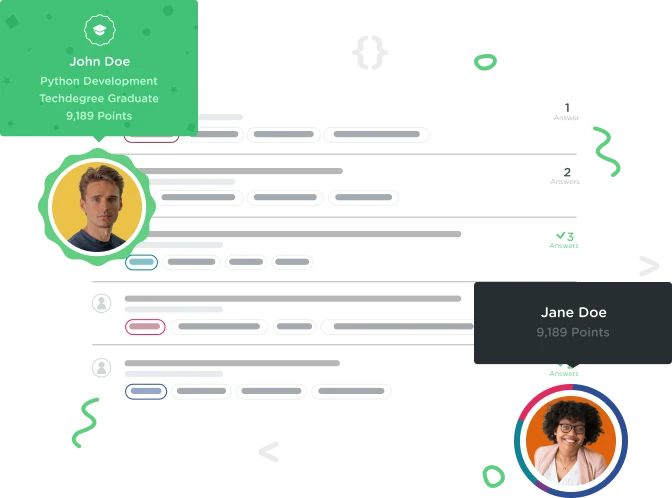

Joel Glovier
3,010 PointsWhen is it helpful to store a function in a variable instead of simply writing a function declaration?
In the video you mention that you can store functions in a variable, although you won't be using that style. Is it just another way of doing things, or is there a particular situation when storing as a variable is better than simply declaring? Can you give an example?
Thanks!
2 Answers
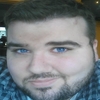
Marcus Parsons
15,719 PointsHey Joel,
This is an interesting question. I personally prefer to write function declarations (not using var keyword) rather than writing function expressions (using var keyword). I think it's easier to read function declarations than it is to read function expressions, and you can declare and use function declarations from anywhere on the page whereas with function expressions, they must be initialized before they are called i.e. higher up on the page than any call that's made to them.
And, as far as speed goes, I actually recently learned that it varies per browser which is fastest. In Chrome, function declarations are many times faster than function expressions. In Firefox, however, the opposite is true! Here is the JS performance test you should run from both Firefox and Chrome: http://jsperf.com/function-declaration-vs-function-expression
//This is valid to use and will execute saying "Hi there!"
myFunc1();
function myFunc1() {
alert("Hi there!");
}
//This is invalid and will give you a type error or say that it is undefined
myFunc2();
var myFunc2 = function () {
alert("Hey there!");
}
//This is valid but it will not execute on either Chrome or Firefox (and more than likely others)
//Because of myFunc2 improper calling
var myFunc3 = function () {
alert("Howdy there!");
}
myFunc3();

Joel Glovier
3,010 PointsMarcus, thanks for your input. Other than browser specific performance, are there times when architecturally it makes more sense to use the keyword versions?
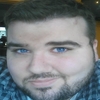
Marcus Parsons
15,719 PointsFrom what I've seen within my own projects and many others, it depends on how you like to write code. To me, it doesn't make sense to use function expressions except in certain cases because they are both obviously fast but declarations can be used anywhere.
One instance where I've written function expressions (and it made sense to do so) is in my ExtraMath.js library I wrote for calculations which you can check out here: https://github.com/marcusparsons/ExtraMath.js
This library utilizes polyfill functions which take the form of function expressions. A polyfill function is a function that either utilizes the function in the compiler if it is present or the custom function created if it is not. Here is an example:
//Math.acosh is an experimental math method that is not available in older browsers and
//is still considered to be experimental due to its lack of browser compatibility. This function allows
//access to this function to older browsers
Math.acosh = Math.acosh || function (arg) {
try {
arg = parseFloat(arg);
if (arg < 1) {
showError("Error: acosh only accepts numbers greater than or equal to 1");
return 0;
}
else {
return round(Math.log(arg + Math.sqrt(arg * arg - 1)), defPrec);
}
}
catch (err) {
showError(errorMsg + err);
}
}
Fernando Seminario
1,976 PointsFernando Seminario
1,976 PointsJust so it is clear... Are you stating that the third example won't work because myFunc2 is invalid and as a result none of the function expressions declared after myFunc2 are valid?
If you take out myFunc2 then myFunc3 will work?